根据上面要求写出代码
时间: 2024-10-12 17:09:08 浏览: 17
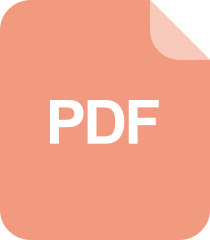
写出健壮的Bash脚本
编写遗传算法来解决上述优化问题是相当复杂的,因为它涉及到了很多关键步骤,包括初始化、适应度评估、选择、交叉和变异。这里我会提供一个基本的Python代码框架,但请注意,这只是一个简化版本,实际应用可能需要更详细的错误处理和参数调整:
```python
import numpy as np
import random
# 定义函数
def fitness_function(x, y):
return 20 + x**2 - 10 * np.cos(2*np.pi*x) + y**2 - 10 * np.cos(2*np.pi*y)
# 遗传算法参数
pop_size = 100 # 种群大小
max_gen = 1000 # 最大世代数
cross_rate = 0.8 # 交叉概率
mutate_rate = 0.1 # 变异概率
elitism = 5 # 精英保留数量
# 初始化种群
population = [(random.uniform(-5, 5), random.uniform(-5, 5)) for _ in range(pop_size)]
for gen in range(max_gen):
# 评估适应度
fitness_values = [fitness_function(*individual) for individual in population]
# 选择
parents = [population[i] for i in sorted(range(pop_size), key=lambda j: fitness_values[j])[:elitism]]
remaining_parents = random.choices(population[elitism:], k=pop_size - elitism)
# 交叉
offspring = []
for _ in range(len(parents)):
parent1, parent2 = random.choice(parents), random.choice(remaining_parents)
if random.random() < cross_rate:
crossover_point = random.randint(1, len(parent1)-1)
child = parent1[:crossover_point] + parent2[crossover_point:]
else:
child = parent1
offspring.append(child)
# 变异
mutated_offspring = [(child[0] + mutate_rate * random.uniform(-1, 1), child[1] + mutate_rate * random.uniform(-1, 1))
if random.random() < mutate_rate else child
for child in offspring]
# 更新种群
population = parents + mutated_offspring
# 返回最佳解
best_solution = population[fitness_values.index(min(fitness_values))]
min_fitness = fitness_function(*best_solution)
print(f"全局最小值:{min_fitness}, 位置:({best_solution[0]}, {best_solution[1]})")
```
请注意,这个代码示例并不保证能找到全局最小值,因为遗传算法的结果依赖于随机性和初始种群的选择。为了得到可靠结果,可能需要多次运行并取平均值。
阅读全文
相关推荐
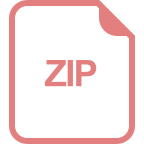
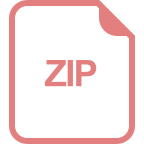















