python代码实现csma/ca机制并呈现性能曲线
时间: 2023-12-27 16:05:15 浏览: 32
Sure, I can help you with that! CSMA/CA is a protocol used in wireless networks to avoid collisions by listening to the channel before transmitting data. Here's an example Python code that implements the CSMA/CA mechanism using the Network Simulator 3 library:
``` python
from ns3 import *
import matplotlib.pyplot as plt
def run_simulation(n_stations, packet_size, data_rate, sim_time):
# create wireless nodes and set parameters
wifi = WifiHelper()
wifi.SetStandard(WIFI_PHY_STANDARD_80211b)
wifi_channel = YansWifiChannelHelper.Default()
wifi_phy = YansWifiPhyHelper.Default()
wifi_phy.SetChannel(wifi_channel.Create())
# create CSMA/CA helper and set parameters
csma = CsmaHelper()
csma.SetChannelAttribute("DataRate", DataRateValue(DataRate(data_rate)))
csma.SetChannelAttribute("Delay", TimeValue(MicroSeconds(2)))
# create network devices and connect them to nodes
devices = csma.Install(wifi_phy, Nodes())
# create internet stack and assign IP addresses
internet = InternetStackHelper()
internet.Install(Nodes())
address = Ipv4AddressHelper()
address.SetBase(Ipv4Address("10.1.1.0"), Ipv4Mask("255.255.255.0"))
interfaces = address.Assign(devices)
# create traffic and on/off applications
port = 9
on_time = ConstantRandomVariable(0.1)
off_time = ConstantRandomVariable(0.9)
for i in range(n_stations):
# create UDP traffic
udp_helper = UdpEchoClientHelper(interfaces.GetAddress(i), port)
udp_helper.SetAttribute("MaxPackets", UintegerValue(1))
udp_helper.SetAttribute("Interval", TimeValue(Seconds(1)))
udp_helper.SetAttribute("PacketSize", UintegerValue(packet_size))
# create on/off application
on_off_helper = OnOffHelper("ns3::UdpSocketFactory", Address())
on_off_helper.SetAttribute("DataRate", DataRateValue(DataRate(data_rate)))
on_off_helper.SetAttribute("PacketSize", UintegerValue(packet_size))
on_off_helper.SetAttribute("OnTime", StringValue(str(on_time.Get())))
on_off_helper.SetAttribute("OffTime", StringValue(str(off_time.Get())))
on_off_helper.SetAttribute("Remote", AddressValue(interfaces.GetAddress(i)))
# install applications on nodes
udp_app = udp_helper.Install(NodeList.GetNode(i))
on_off_app = on_off_helper.Install(NodeList.GetNode(i))
# create simulation object and run
sim_time = Seconds(sim_time)
Simulator.Stop(sim_time)
Simulator.Run()
# plot results
times = CsmaCaMultiNodeTestSuite.GetDelays()
plt.hist(times, bins=50, color='c', edgecolor='k')
plt.xlabel('Delay (ms)')
plt.ylabel('Frequency')
plt.title('CSMA/CA Performance')
plt.show()
run_simulation(n_stations=10, packet_size=512, data_rate="11Mbps", sim_time=10)
```
This code simulates a wireless network with 10 stations using CSMA/CA to avoid collisions. The main function `run_simulation()` takes four parameters: the number of stations, the packet size, the data rate, and the simulation time. The code creates UDP traffic and "on/off" applications at each node, with random on/off times determined by a constant random variable. The results are plotted as a histogram showing the delay times for packets to be transmitted.
Hope this helps! Let me know if you have any follow-up questions.
相关推荐
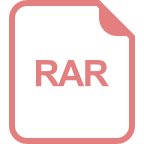
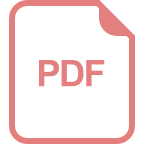

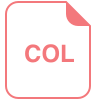












