C语言实现飞机大战游戏
时间: 2023-11-16 22:06:37 浏览: 41
飞机大战游戏是一款经典的飞行射击游戏,可以用C语言实现。下面是一个简单的示例代码,可以用来实现基本的游戏功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <Windows.h>
#define WIDTH 80
#define HEIGHT 30
#define MAX_ENEMIES 5
#define ENEMY_SPEED 2
typedef struct {
int x, y, speed, alive;
} Enemy;
typedef struct {
int x, y;
} Bullet;
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_ship(int x, int y) {
gotoxy(x, y);
printf(" /\\");
gotoxy(x, y+1);
printf("<###>");
gotoxy(x, y+2);
printf(" \\/");
}
void draw_bullet(Bullet bullet) {
gotoxy(bullet.x, bullet.y);
printf("|");
}
void draw_enemy(Enemy enemy) {
if(enemy.alive) {
gotoxy(enemy.x, enemy.y);
printf("^");
}
}
void update_bullet(Bullet *bullet) {
bullet->y--;
}
void update_enemy(Enemy *enemy) {
enemy->y += enemy->speed;
if(enemy->y >= HEIGHT) {
enemy->alive = 0;
}
}
void fire_bullet(Bullet bullets[], int *num_bullets, int x, int y) {
if(*num_bullets < 10) {
bullets[*num_bullets].x = x+2;
bullets[*num_bullets].y = y-1;
(*num_bullets)++;
}
}
int main() {
int ship_x = WIDTH/2-1;
int ship_y = HEIGHT-4;
int num_bullets = 0;
Bullet bullets[10];
Enemy enemies[MAX_ENEMIES];
int num_enemies = 0;
int score = 0;
int game_over = 0;
int i, j;
for(i=0; i<MAX_ENEMIES; i++) {
enemies[i].alive = 0;
}
draw_ship(ship_x, ship_y);
while(!game_over) {
if(kbhit()) {
char ch = getch();
if(ch == 'a' && ship_x > 0) {
ship_x--;
}
else if(ch == 'd' && ship_x < WIDTH-5) {
ship_x++;
}
else if(ch == ' ') {
fire_bullet(bullets, &num_bullets, ship_x, ship_y);
}
}
for(i=0; i<num_bullets; i++) {
update_bullet(&bullets[i]);
if(bullets[i].y < 0) {
bullets[i] = bullets[--num_bullets];
}
}
for(i=0; i<num_enemies; i++) {
update_enemy(&enemies[i]);
if(enemies[i].alive && enemies[i].x == ship_x+2 && enemies[i].y == ship_y-1) {
game_over = 1;
break;
}
for(j=0; j<num_bullets; j++) {
if(enemies[i].alive && enemies[i].x == bullets[j].x && enemies[i].y == bullets[j].y) {
enemies[i].alive = 0;
bullets[j] = bullets[--num_bullets];
score++;
break;
}
}
}
if(num_enemies < MAX_ENEMIES && rand()%100 < 10) {
for(i=0; i<MAX_ENEMIES; i++) {
if(!enemies[i].alive) {
enemies[i].x = rand()%(WIDTH-2)+1;
enemies[i].y = 0;
enemies[i].speed = ENEMY_SPEED;
enemies[i].alive = 1;
num_enemies++;
break;
}
}
}
system("cls");
for(i=0; i<WIDTH; i++) {
gotoxy(i, 0);
printf("-");
gotoxy(i, HEIGHT-1);
printf("-");
}
for(i=1; i<HEIGHT-1; i++) {
gotoxy(0, i);
printf("|");
gotoxy(WIDTH-1, i);
printf("|");
}
draw_ship(ship_x, ship_y);
for(i=0; i<num_bullets; i++) {
draw_bullet(bullets[i]);
}
for(i=0; i<num_enemies; i++) {
draw_enemy(enemies[i]);
}
gotoxy(WIDTH+2, 2);
printf("Score: %d", score);
Sleep(50);
}
system("cls");
gotoxy(WIDTH/2-5, HEIGHT/2-1);
printf("Game Over!");
gotoxy(WIDTH/2-7, HEIGHT/2);
printf("Final Score: %d", score);
gotoxy(0, HEIGHT);
return 0;
}
```
该代码使用了Windows系统的控制台API,因此只能在Windows系统上运行。如果要在其他系统上运行,需要使用其他的图形库或框架。代码中使用了结构体来表示敌人和子弹对象,使用了循环来更新敌人和子弹的位置,使用了随机数来生成敌人对象。当敌人与玩家的飞机相撞或者子弹打中敌人时,会更新分数。当敌人撞到底部边界时,会消失。当玩家的飞机被敌人撞到时,游戏结束,显示最终分数。
相关推荐
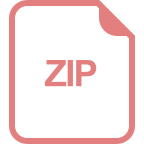
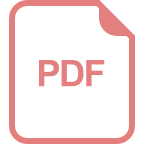














