request for member ‘get_future’ in ‘task’, which is of non-class type ‘std::packaged_task<int(__gnu_cxx::__normal_iterator<int*, std::vector<int> >, __gnu_cxx::__normal_iterator<int*, std::vector<int> >)>(accumulate_block<__gnu_cxx::__normal_iterator<int*, std::vector<int> >, int> (*)())’ futures[i]=task.get_future();
时间: 2024-03-15 11:44:02 浏览: 99
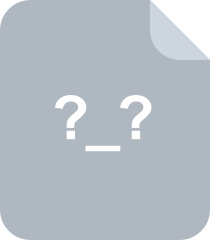
C++11之std::future以及async函数、promise类和packaged_task类结构图
这个错误的原因是`task`变量的类型不是一个类类型,因此不能调用`get_future()`函数。在这里,`task`的类型是一个函数类型,它返回一个`std::packaged_task`对象,而不是一个`std::packaged_task`对象本身。
要解决这个错误,需要在创建`std::packaged_task`对象时使用函数类型的别名或函数指针类型。例如,如果要使用函数类型别名`Func`来定义`std::packaged_task`对象,可以这样写:
```c++
using Func = int(std::vector<int>::iterator, std::vector<int>::iterator);
std::packaged_task<Func> task(accumulate_block<int>);
```
或者,使用函数指针类型`FuncPtr`定义`std::packaged_task`对象,可以这样写:
```c++
using FuncPtr = int(*)(std::vector<int>::iterator, std::vector<int>::iterator);
std::packaged_task<FuncPtr> task(&accumulate_block<int>);
```
这样可以确保`task`的类型为`std::packaged_task`,从而可以调用`get_future()`函数获取与任务关联的`std::future`对象。
阅读全文
相关推荐
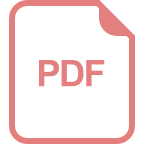


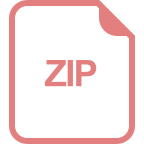
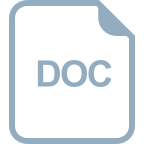
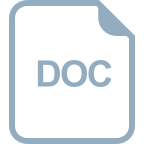
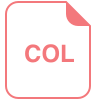







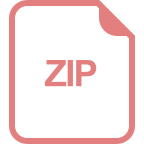
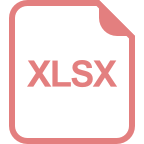