一个100*100的网格,里面包含随机0-100数字,设计一个算法,找到所有峰值,并输出网格下标C++
时间: 2024-03-21 14:39:13 浏览: 48
好的,下面是一个C++实现的分治算法,用于在一个100*100的网格中找到所有峰值,并输出网格下标:
```c++
#include <iostream>
#include <vector>
using namespace std;
void find_peak(vector<vector<int>>& matrix, int start_col, int end_col, int row, vector<pair<int, int>>& result) {
int mid_col = (start_col + end_col) / 2;
int max_row = row;
int max_val = matrix[row][mid_col];
for (int i = 0; i < matrix.size(); i++) {
if (matrix[i][mid_col] > max_val) {
max_val = matrix[i][mid_col];
max_row = i;
}
}
if ((mid_col == start_col || matrix[max_row][mid_col-1] <= max_val) && (mid_col == end_col || matrix[max_row][mid_col+1] <= max_val)) {
result.push_back(make_pair(max_row, mid_col));
} else if (mid_col > start_col && matrix[max_row][mid_col-1] > max_val) {
find_peak(matrix, start_col, mid_col-1, max_row, result);
}
if (mid_col < end_col && matrix[max_row][mid_col+1] > max_val) {
find_peak(matrix, mid_col+1, end_col, max_row, result);
}
}
vector<pair<int, int>> find_all_peaks(vector<vector<int>>& matrix) {
vector<pair<int, int>> result;
find_peak(matrix, 0, matrix[0].size()-1, 0, result);
return result;
}
int main() {
vector<vector<int>> matrix(100, vector<int>(100));
for (int i = 0; i < 100; i++) {
for (int j = 0; j < 100; j++) {
matrix[i][j] = rand() % 101;
}
}
vector<pair<int, int>> result = find_all_peaks(matrix);
for (int i = 0; i < result.size(); i++) {
cout << "Peak found at (" << result[i].first << ", " << result[i].second << ")" << endl;
}
return 0;
}
```
这个算法的时间复杂度也是O(m log n),其中m是矩阵的行数,n是矩阵的列数。
阅读全文
相关推荐
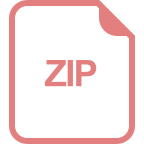
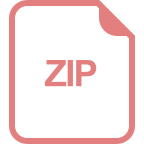
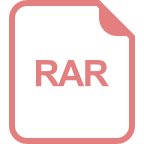
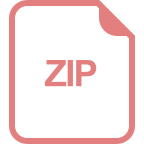
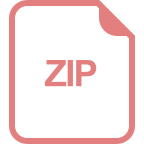
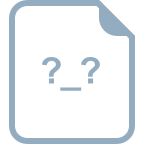
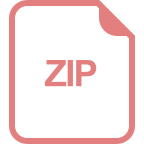
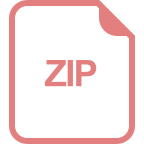
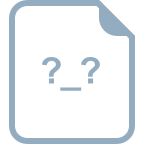
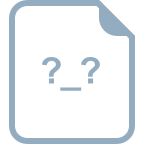
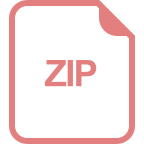
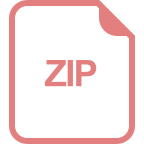
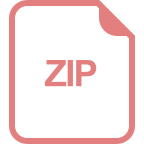
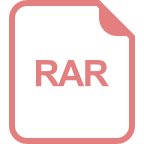
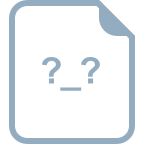