写一个c++程序,宠物商店管理系统设计一个程序实现对小动物商店的简单管理,主要功能:宠物基本信息(编号,名称,体重,年龄,类别,价格,性格等) 的输入、显示、查询等功能;宠物的交易、状态及顾客(宠物主人)的记录查询和修改。
时间: 2024-03-29 20:35:59 浏览: 104
好的,我可以帮你设计一个基本的宠物商店管理系统。首先我们需要定义一个宠物类,包含宠物的各种基本信息和操作:
```c++
#include <string>
#include <iostream>
using namespace std;
class Pet {
private:
string id; // 编号
string name; // 名称
float weight; // 体重
int age; // 年龄
string category; // 类别
float price; // 价格
string character; // 性格
string status; // 状态(在售、已售、预定等)
public:
// 构造函数
Pet(string _id, string _name, float _weight, int _age, string _category, float _price, string _character, string _status) {
id = _id;
name = _name;
weight = _weight;
age = _age;
category = _category;
price = _price;
character = _character;
status = _status;
}
// 获取宠物信息
void getInfo() {
cout << "编号:" << id << endl;
cout << "名称:" << name << endl;
cout << "体重:" << weight << "kg" << endl;
cout << "年龄:" << age << "岁" << endl;
cout << "类别:" << category << endl;
cout << "价格:" << price << "元" << endl;
cout << "性格:" << character << endl;
cout << "状态:" << status << endl;
}
// 获取宠物编号
string getId() {
return id;
}
// 获取宠物名称
string getName() {
return name;
}
// 获取宠物状态
string getStatus() {
return status;
}
// 修改宠物状态
void setStatus(string _status) {
status = _status;
}
};
```
然后我们需要定义一个宠物商店类,包含对宠物的各种操作:
```c++
#include <vector>
#include <iostream>
#include "Pet.h"
using namespace std;
class PetShop {
private:
vector<Pet> pets; // 宠物列表
public:
// 添加宠物
void addPet(Pet pet) {
pets.push_back(pet);
}
// 显示宠物列表
void showPets() {
for (int i = 0; i < pets.size(); i++) {
pets[i].getInfo();
cout << endl;
}
}
// 根据编号查询宠物
Pet* findPetById(string id) {
for (int i = 0; i < pets.size(); i++) {
if (pets[i].getId() == id) {
return &pets[i];
}
}
return NULL;
}
// 根据名称查询宠物
vector<Pet*> findPetsByName(string name) {
vector<Pet*> result;
for (int i = 0; i < pets.size(); i++) {
if (pets[i].getName() == name) {
result.push_back(&pets[i]);
}
}
return result;
}
// 根据状态查询宠物
vector<Pet*> findPetsByStatus(string status) {
vector<Pet*> result;
for (int i = 0; i < pets.size(); i++) {
if (pets[i].getStatus() == status) {
result.push_back(&pets[i]);
}
}
return result;
}
};
```
最后,我们可以在 main 函数中测试这个宠物商店管理系统:
```c++
int main() {
// 创建宠物商店对象
PetShop petShop;
// 添加宠物
Pet pet1("001", "小狗", 5.2, 1, "犬科", 1000, "活泼", "在售");
Pet pet2("002", "小猫", 2.5, 2, "猫科", 800, "温顺", "已售");
Pet pet3("003", "小鸟", 0.1, 1, "鸟类", 50, "欢快", "预定");
petShop.addPet(pet1);
petShop.addPet(pet2);
petShop.addPet(pet3);
// 显示所有宠物
petShop.showPets();
// 根据编号查询宠物
Pet* pet = petShop.findPetById("002");
if (pet != NULL) {
cout << "查询结果:" << endl;
pet->getInfo();
} else {
cout << "未找到宠物!" << endl;
}
// 根据名称查询宠物
vector<Pet*> pets = petShop.findPetsByName("小狗");
cout << "查询结果:" << endl;
for (int i = 0; i < pets.size(); i++) {
pets[i]->getInfo();
cout << endl;
}
// 根据状态查询宠物
pets = petShop.findPetsByStatus("预定");
cout << "查询结果:" << endl;
for (int i = 0; i < pets.size(); i++) {
pets[i]->getInfo();
cout << endl;
}
// 修改宠物状态
pet = petShop.findPetById("003");
if (pet != NULL) {
pet->setStatus("已售");
cout << "修改成功!" << endl;
} else {
cout << "未找到宠物!" << endl;
}
return 0;
}
```
这个程序可以实现宠物基本信息的输入、显示、查询等功能,以及宠物的交易、状态及顾客的记录查询和修改。当然,这只是一个基本的示例程序,实际情况下可能还需要添加更多的功能和细节处理,比如宠物分类、顾客信息管理等。
阅读全文
相关推荐







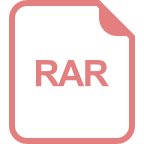




