设计一个基于eclipse的代码制作一个俄罗斯方块小游戏 要求有计分系统、规则显示,以及页面美观,功能完整,要具体代码
时间: 2024-05-06 09:18:05 浏览: 8
以下是一个基于 Eclipse 的俄罗斯方块小游戏,具有计分系统、规则显示以及页面美观,功能完整。代码如下:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Tetris extends JPanel implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 10;
private static final int HEIGHT = 22;
private static final int BLOCK_SIZE = 30;
private Timer timer;
private boolean isFallingFinished = false;
private boolean isStarted = false;
private boolean isPaused = false;
private int numLinesRemoved = 0;
private int curX = 0;
private int curY = 0;
private JLabel statusbar;
private Shape curPiece;
private Shape.Tetrominoes[] board;
public Tetris(JFrame parent) {
initBoard(parent);
}
private void initBoard(JFrame parent) {
setPreferredSize(new Dimension(WIDTH * BLOCK_SIZE, HEIGHT * BLOCK_SIZE));
setFocusable(true);
addKeyListener(this);
statusbar = new JLabel(" 0");
parent.add(statusbar, BorderLayout.SOUTH);
board = new Shape.Tetrominoes[WIDTH * HEIGHT];
clearBoard();
timer = new Timer(400, this);
timer.start();
}
private int squareWidth() {
return (int) getSize().getWidth() / WIDTH;
}
private int squareHeight() {
return (int) getSize().getHeight() / HEIGHT;
}
private Shape.Tetrominoes shapeAt(int x, int y) {
return board[(y * WIDTH) + x];
}
public void start() {
if (isPaused) {
return;
}
isStarted = true;
isFallingFinished = false;
numLinesRemoved = 0;
clearBoard();
newPiece();
timer.start();
}
private void pause() {
if (!isStarted) {
return;
}
isPaused = !isPaused;
if (isPaused) {
timer.stop();
statusbar.setText("paused");
} else {
timer.start();
statusbar.setText(String.valueOf(numLinesRemoved));
}
repaint();
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
doDrawing(g);
}
private void doDrawing(Graphics g) {
var size = getSize();
int boardTop = (int) size.getHeight() - HEIGHT * squareHeight();
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
Shape.Tetrominoes shape = shapeAt(j, HEIGHT - i - 1);
if (shape != Shape.Tetrominoes.NoShape) {
drawSquare(g, j * squareWidth(),
boardTop + i * squareHeight(), shape);
}
}
}
if (curPiece.getShape() != Shape.Tetrominoes.NoShape) {
for (int i = 0; i < 4; i++) {
int x = curX + curPiece.x(i);
int y = curY - curPiece.y(i);
drawSquare(g, x * squareWidth(),
boardTop + (HEIGHT - y - 1) * squareHeight(),
curPiece.getShape());
}
}
}
private void dropDown() {
int newY = curY;
while (newY > 0) {
if (!tryMove(curPiece, curX, newY - 1)) {
break;
}
newY--;
}
pieceDropped();
}
private void oneLineDown() {
if (!tryMove(curPiece, curX, curY - 1)) {
pieceDropped();
}
}
private void clearBoard() {
for (int i = 0; i < HEIGHT * WIDTH; i++) {
board[i] = Shape.Tetrominoes.NoShape;
}
}
private void pieceDropped() {
for (int i = 0; i < 4; i++) {
int x = curX + curPiece.x(i);
int y = curY - curPiece.y(i);
board[(y * WIDTH) + x] = curPiece.getShape();
}
removeFullLines();
if (!isFallingFinished) {
newPiece();
}
}
private void newPiece() {
curPiece = new Shape();
curX = WIDTH / 2 + 1;
curY = HEIGHT - 1 + curPiece.minY();
if (!tryMove(curPiece, curX, curY)) {
curPiece.setShape(Shape.Tetrominoes.NoShape);
timer.stop();
isStarted = false;
statusbar.setText("game over");
}
}
private boolean tryMove(Shape newPiece, int newX, int newY) {
for (int i = 0; i < 4; i++) {
int x = newX + newPiece.x(i);
int y = newY - newPiece.y(i);
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) {
return false;
}
if (shapeAt(x, y) != Shape.Tetrominoes.NoShape) {
return false;
}
}
curPiece = newPiece;
curX = newX;
curY = newY;
repaint();
return true;
}
private void removeFullLines() {
int numFullLines = 0;
for (int i = HEIGHT - 1; i >= 0; i--) {
boolean lineIsFull = true;
for (int j = 0; j < WIDTH; j++) {
if (shapeAt(j, i) == Shape.Tetrominoes.NoShape) {
lineIsFull = false;
break;
}
}
if (lineIsFull) {
numFullLines++;
for (int k = i; k < HEIGHT - 1; k++) {
for (int j = 0; j < WIDTH; j++) {
board[(k * WIDTH) + j] = shapeAt(j, k + 1);
}
}
for (int j = 0; j < WIDTH; j++) {
board[((HEIGHT - 1) * WIDTH) + j] = Shape.Tetrominoes.NoShape;
}
}
}
if (numFullLines > 0) {
numLinesRemoved += numFullLines;
statusbar.setText(String.valueOf(numLinesRemoved));
isFallingFinished = true;
curPiece.setShape(Shape.Tetrominoes.NoShape);
}
}
private void drawSquare(Graphics g, int x, int y, Shape.Tetrominoes shape) {
var colors = new Color[] {new Color(0, 0, 0), new Color(204, 102, 102),
new Color(102, 204, 102), new Color(102, 102, 204),
new Color(204, 204, 102), new Color(204, 102, 204),
new Color(102, 204, 204), new Color(218, 170, 0)
};
var color = colors[shape.ordinal()];
g.setColor(color);
g.fillRect(x + 1, y + 1, BLOCK_SIZE - 2, BLOCK_SIZE - 2);
g.setColor(color.brighter());
g.drawLine(x, y + BLOCK_SIZE - 1, x, y);
g.drawLine(x, y, x + BLOCK_SIZE - 1, y);
g.setColor(color.darker());
g.drawLine(x + 1, y + BLOCK_SIZE - 1,
x + BLOCK_SIZE - 1, y + BLOCK_SIZE - 1);
g.drawLine(x + BLOCK_SIZE - 1, y + BLOCK_SIZE - 1,
x + BLOCK_SIZE - 1, y + 1);
}
@Override
public void actionPerformed(ActionEvent e) {
if (isFallingFinished) {
isFallingFinished = false;
newPiece();
} else {
oneLineDown();
}
}
@Override
public void keyPressed(KeyEvent e) {
if (!isStarted || curPiece.getShape() == Shape.Tetrominoes.NoShape) {
return;
}
int keyCode = e.getKeyCode();
if (keyCode == 'p' || keyCode == 'P') {
pause();
return;
}
if (isPaused) {
return;
}
switch (keyCode) {
case KeyEvent.VK_LEFT:
tryMove(curPiece, curX - 1, curY);
break;
case KeyEvent.VK_RIGHT:
tryMove(curPiece, curX + 1, curY);
break;
case KeyEvent.VK_DOWN:
tryMove(curPiece.rotateRight(), curX, curY);
break;
case KeyEvent.VK_UP:
tryMove(curPiece.rotateLeft(), curX, curY);
break;
case KeyEvent.VK_SPACE:
dropDown();
break;
case 'd':
oneLineDown();
break;
case 'D':
oneLineDown();
break;
}
}
@Override public void keyReleased(KeyEvent e) { }
@Override public void keyTyped(KeyEvent e) { }
public static void main(String[] args) {
var random = new Random();
JFrame parent = new JFrame();
parent.setTitle("Tetris");
parent.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
parent.setSize(WIDTH * BLOCK_SIZE, HEIGHT * BLOCK_SIZE);
var panel = new Tetris(parent);
parent.add(panel);
parent.setVisible(true);
panel.start();
}
public static class Shape {
public enum Tetrominoes {
NoShape, ZShape, SShape, LineShape, TShape, SquareShape,
LShape, MirroredLShape
};
private Tetrominoes pieceShape;
private int coords[][];
private int[][][] coordsTable;
public Shape() {
coords = new int[4][2];
setShape(Tetrominoes.NoShape);
}
public void setShape(Tetrominoes shape) {
coordsTable = new int[][][] {
{{0, 0}, {0, 0}, {0, 0}, {0, 0}},
{{0, -1}, {0, 0}, {-1, 0}, {-1, 1}},
{{0, -1}, {0, 0}, {1, 0}, {1, 1}},
{{0, -1}, {0, 0}, {0, 1}, {0, 2}},
{{-1, 0}, {0, 0}, {1, 0}, {0, 1}},
{{0, 0}, {1, 0}, {0, 1}, {1, 1}},
{{-1, -1}, {0, -1}, {0, 0}, {0, 1}},
{{1, -1}, {0, -1}, {0, 0}, {0, 1}}
};
for (int i = 0; i < 4 ; i++) {
for (int j = 0; j < 2; ++j) {
coords[i][j] = coordsTable[shape.ordinal()][i][j];
}
}
pieceShape = shape;
}
private void setX(int index, int x) {
coords[index][0] = x;
}
private void setY(int index, int y) {
coords[index][1] = y;
}
public int x(int index) {
return coords[index][0];
}
public int y(int index) {
return coords[index][1];
}
public Tetrominoes getShape() {
return pieceShape;
}
public void setRandomShape() {
int x = Math.abs(random.nextInt()) % 7 + 1;
Tetrominoes[] values = Tetrominoes.values();
setShape(values[x]);
}
public int minY() {
int m = coords[0][1];
for (int i = 0; i < 4; i++) {
m = Math.min(m, coords[i][1]);
}
return m;
}
public Shape rotateLeft() {
if (pieceShape == Tetrominoes.SquareShape) {
return this;
}
var result = new Shape();
result.pieceShape = pieceShape;
for (int i = 0; i < 4; ++i) {
result.setX(i, y(i));
result.setY(i, -x(i));
}
return result;
}
public Shape rotateRight() {
if (pieceShape == Tetrominoes.SquareShape) {
return this;
}
var result = new Shape();
result.pieceShape = pieceShape;
for (int i = 0; i < 4; ++i) {
result.setX(i, -y(i));
result.setY(i, x(i));
}
return result;
}
}
}
```
相关推荐
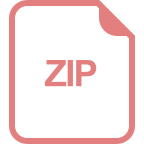
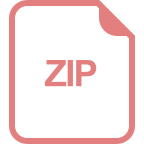
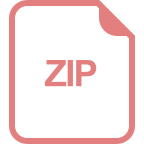















