std::string分割逗号
时间: 2024-05-27 19:07:26 浏览: 16
可以使用C++标准库中的stringstream和getline函数来实现std::string的逗号分割,具体实现代码如下:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
using namespace std;
vector<string> split(const string &s, char delim) {
vector<string> result;
stringstream ss(s);
string item;
while (getline(ss, item, delim)) {
result.push_back(item);
}
return result;
}
int main() {
string str = "C++,Java,Python,C#,JavaScript";
vector<string> vec = split(str, ',');
for (const auto &s : vec) {
cout << s << endl;
}
return 0;
}
```
以上代码将字符串str按照逗号分割成一个字符串数组vec,可以方便地遍历输出分割后的结果。如果需要分割其他字符,只需要将split函数中的参数delim改为对应字符即可。
相关问题
std::string分割
std::string分割是将一个字符串分割成多个子串的操作。在C++中,可以通过使用std::stringstream或者使用循环遍历字符串来实现分割。以下是两种常用的分割方法:
方法1:使用std::stringstream
可以使用std::stringstream将字符串转换为流,并使用getline()函数从流中逐行提取子串。首先,将待分割的字符串放入std::stringstream中,然后使用getline()函数从流中逐行读取子串,并将其存储在一个容器中。
示例代码如下:
```cpp
std::string str = "Hello,World,This,is,a,string";
std::stringstream ss(str);
std::string token;
std::vector<std::string> tokens;
while (getline(ss, token, ',')) {
tokens.push_back(token);
}
```
以上代码将字符串"Hello,World,This,is,a,string"按照逗号分割成多个子串,并将子串存储在名为tokens的std::vector容器中。
方法2:使用循环遍历字符串
可以使用循环遍历字符串的方式,逐个字符查找分割符号,并将分割符号前的子串存储在一个容器中。
示例代码如下:
```cpp
std::string str = "Hello,World,This,is,a,string";
std::string delimiter = ",";
std::vector<std::string> tokens;
size_t pos = 0;
size_t prev = 0;
while ((pos = str.find(delimiter, prev)) != std::string::npos) {
std::string token = str.substr(prev, pos - prev);
tokens.push_back(token);
prev = pos + 1;
}
// 处理最后一个子串
std::string token = str.substr(prev);
tokens.push_back(token);
```
以上代码将字符串"Hello,World,This,is,a,string"按照逗号分割成多个子串,并将子串存储在名为tokens的std::vector容器中。
这是std::string分割的两种常用方法,你可以根据具体的需求选择合适的方法进行分割。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C++字符串操作案例(字符串拼接、替换、分割、类型转换、截取)](https://blog.csdn.net/a486259/article/details/124966131)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
std::string 字符串分割 c++代码
### 回答1:
你可以使用 C++ 的 string 类的 find 和 substr 函数来分割 std::string 字符串。
例如,如果你想要将字符串按照逗号分割,你可以使用以下代码:
```
#include <iostream>
#include <string>
#include <vector>
std::vector<std::string> split(const std::string& str, char delimiter) {
std::vector<std::string> tokens;
std::string token;
std::istringstream tokenStream(str);
while (std::getline(tokenStream, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
std::string str = "apple,banana,orange";
char delimiter = ',';
std::vector<std::string> tokens = split(str, delimiter);
for (const std::string& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
```
输出结果为:
apple
banana
orange
### 回答2:
std::string 字符串分割的 c++ 代码可以使用以下方式实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <sstream>
std::vector<std::string> splitString(const std::string& str, char delimiter) {
std::vector<std::string> result;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, delimiter)) {
result.push_back(token);
}
return result;
}
int main() {
std::string str = "Hello,World,This,is,a,test";
std::vector<std::string> words = splitString(str, ',');
for (const std::string& word : words) {
std::cout << word << std::endl;
}
return 0;
}
```
以上代码中的 splitString 函数接受两个参数,一个是要分割的字符串 str,另一个是分隔符 delimiter。通过使用 std::stringstream 类来进行字符串的分割操作。首先将要分割的字符串 str 传入到 stringstream 对象 ss 中,然后使用 getline 函数从 ss 中按照指定的分隔符 delimiter 提取每个子字符串,并将其存储到 result 向量中。最后返回存储了分割结果的向量。
在 main 函数中,我们可以看到示例代码将一个包含逗号的字符串进行了分割。逗号是作为分隔符进行字符串的分割。分割结果存储在向量 words 中,并通过循环将每个子字符串输出到控制台上。
### 回答3:
要实现字符串分割的c代码,可以使用标准库中的std::string类及其成员函数和标准库中的string流stringstream来实现。以下是一个示例代码:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
std::vector<std::string> splitString(const std::string& input, char delimiter) {
std::istringstream iss(input);
std::vector<std::string> result;
std::string segment;
while (std::getline(iss, segment, delimiter)) {
result.push_back(segment);
}
return result;
}
int main() {
std::string input = "Hello,World,This,is,a,string";
char delimiter = ',';
std::vector<std::string> substrings = splitString(input, delimiter);
for (const std::string& substring : substrings) {
std::cout << substring << std::endl;
}
return 0;
}
```
以上代码中,splitString函数接受两个参数,一个是输入字符串input,另一个是分割符delimiter。函数内部使用std::istringstream将input包装成流以便逐段读取。然后通过std::getline函数的第三个参数delimiter指定每段的分割符,并将每个分段segment存入vector容器result中。最后,函数返回分割的结果。
在主函数中,定义了一个输入字符串input和一个分割符delimiter,并通过调用splitString函数将input按delimiter进行分割。然后,通过遍历substrings容器输出分割后的子串。
以上是一个基本的字符串分割c代码示例,通过使用std::string类和string流stringstream,可以方便地实现字符串的分割功能。
相关推荐
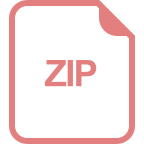
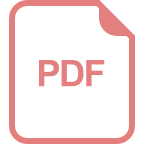
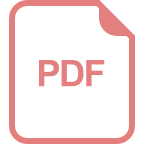












