java实现hbase表查询
时间: 2024-11-05 20:23:55 浏览: 20
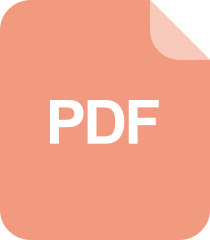
hbase分页查询实现.pdf
在Java中,HBase是一个基于列族(Column Family)的大数据NoSQL数据库,它通常用于处理大规模的数据存储。如果你想要查询HBase表,你可以通过HBase的官方API `HBaseAdmin` 和 `HTable` 来实现。以下是基本步骤:
1. **添加依赖**:
首先,你需要在你的项目中添加HBase的依赖。如果你使用Maven,可以在pom.xml文件中加入相应的依赖。
```xml
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>YOUR_HBASE_VERSION</version>
</dependency>
```
2. **创建连接**:
创建一个`HBaseConfiguration`对象,并配置连接到HBase服务器的相关信息。
```java
HBaseConfiguration config = HBaseConfiguration.create();
config.set("hbase.zookeeper.quorum", "zookeeper_host1,zookeeper_host2,...");
config.set("hbase.zookeeper.property.clientPort", "2181");
```
3. **创建表或检查是否存在**:
使用`HBaseAdmin`实例来创建表或检查表是否存在。
```java
HBaseAdmin admin = new HBaseAdmin(config);
if (!admin.tableExists("your_table_name")) {
// 表不存在,创建表
TableName tableName = TableName.valueOf("your_table_name");
byte[] columnFamilyName = Bytes.toBytes("cf"); // 列族名
admin.createTable(tableName, new byte[][] {columnFamilyName});
}
admin.close(); // 关闭连接
```
4. **执行查询**:
使用`HTable`实例执行扫描操作,例如获取所有行或特定范围的数据。
```java
HTable table = new HTable(config, "your_table_name");
Scan scan = new Scan();
scan.addFamily(Bytes.toBytes("cf")); // 包含指定列族的扫描
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
byte[] rowKey = result.getRow();
List<Cell> cells = result.listCells(); // 获取该行的所有单元格
for (Cell cell : cells) {
byte[] qualifier = cell.getQualifierArray();
byte[] value = cell.getValueArray();
String colFamily = Bytes.toString(qualifier); // 列族
String colQualifier = Bytes.toString(qualifier); // 列名
String data = Bytes.toString(value); // 数据值
System.out.println("Row Key: " + Bytes.toString(rowKey) +
", Column Family: " + colFamily +
", Qualifier: " + colQualifier +
", Value: " + data);
}
}
scanner.close(); // 关闭扫描器
table.close(); // 关闭表连接
```
阅读全文
相关推荐
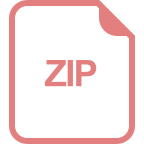
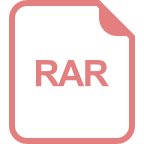
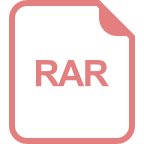
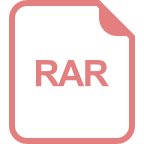
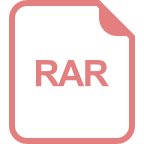
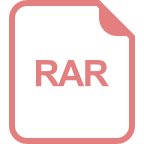
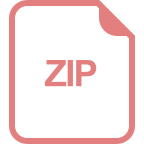
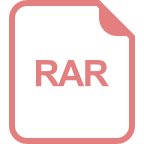
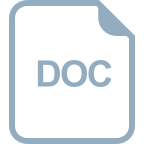
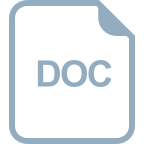
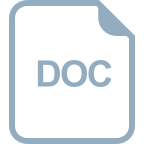
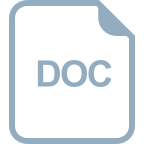


