dataframe.map
时间: 2024-04-29 21:18:33 浏览: 19
pandas中的DataFrame对象拥有一个名为map()的方法,它可以被用于对DataFrame对象中的每个元素进行映射操作。map()方法接受一个函数作为参数,并将其应用于DataFrame中的每个元素,最终返回一个新的Series对象。这个新的Series对象的大小与原来的DataFrame相同。
下面是一个例子,它展示了如何使用map()方法将DataFrame中的每个元素加1:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
def add_one(x):
return x + 1
result = df.map(add_one)
print(result)
```
输出:
```
A B
0 2 5
1 3 6
2 4 7
```
请注意,map()方法不会修改原始DataFrame,而是返回一个新的Series对象。如果您想修改原始DataFrame,请使用inplace=True参数。
相关问题
dataframe.map()执行函数
在 Pandas 中,DataFrame 的 map() 方法可以将一个函数应用于 DataFrame 中每个元素,返回一个新的 DataFrame,其中每个元素都被函数处理后的结果所替换。 map() 方法的常见用法是将一个列中的所有元素进行转换或应用一个函数。
下面是一个示例,说明如何使用 map() 方法将 DataFrame 中的某一列中的所有元素都转换为大写字母:
``` python
import pandas as pd
# 创建 DataFrame
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]})
# 将 name 列中的元素转换为大写字母
df['name'] = df['name'].map(lambda x: x.upper())
# 显示 DataFrame
print(df)
```
输出结果:
```
name age
0 ALICE 25
1 BOB 30
2 CHARLIE 35
```
在上面的示例中,我们使用了 lambda 函数来将 name 列中的所有元素都转换为大写字母。如果你想使用其他函数,只需要将函数名传递给 map() 方法即可。
dataframe转map
将DataFrame转换为Map的方法,可以使用Java 8的Stream API中的collect方法。例如,假设有以下DataFrame:
```
+---+-------+--------+
|id | name | age |
+---+-------+--------+
| 1 | Alice | 25 |
| 2 | Bob | 30 |
| 3 | John | 35 |
+---+-------+--------+
```
想要将DataFrame转换为Map,其中id作为键,每一行作为值,可以使用以下代码:
```java
Map<Integer, Row> map = dataframe
.toJavaRDD()
.collectAsMap()
.entrySet()
.stream()
.collect(Collectors.toMap(
e -> (Integer) e.getKey(),
e -> (Row) e.getValue()
));
```
这将生成一个Map,其中键是id,值是对应行的Row对象。
相关推荐
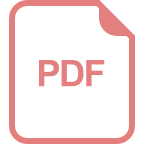
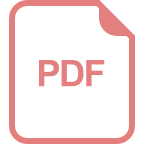
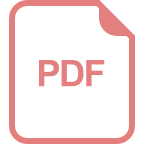
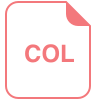











