如何用C++编写一个算法来绘制具有指定间距的折线,折线形状比较复杂,支持向左和向右扩展?
时间: 2024-11-29 20:35:34 浏览: 3
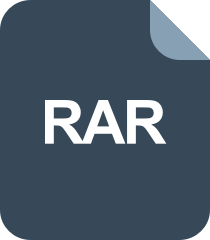
直线和圆弧绘制算法实现.rar

要在C++中编写一个算法来绘制复杂的折线,你可以使用基本的数据结构如数组、列表或向量来存储折线的点,然后遍历这些点并使用绘图函数连接它们。这里是一个简单的示例,假设我们有一个二维点结构`Point`,以及一个函数`drawLine`来画一条直线:
```cpp
#include <vector>
struct Point {
int x;
int y;
};
void drawLine(const Point& start, const Point& end, int spacing) {
if (start.x == end.x) { // 平行于x轴的线
for (int i = start.y; i <= end.y; i += spacing) {
std::cout << "(" << start.x << ", " << i << ")";
if (i != end.y) {
std::cout << " -> ";
}
}
std::cout << std::endl;
} else if (start.y == end.y) { // 平行于y轴的线
for (int i = start.x; i <= end.x; i += spacing) {
std::cout << "(" << i << ", " << start.y << ")";
if (i != end.x) {
std::cout << " -> ";
}
}
std::cout << std::endl;
} else { // 斜线
int dx = end.x - start.x;
int dy = end.y - start.y;
int steepness = std::abs(dy) / std::abs(dx); // 计算斜率
bool increasingY = dy > 0;
for (int i = start.x; ; i += spacing * steepness) {
int yi = start.y + ((increasingY && i <= end.x) || (!increasingY && i >= end.x)) ? steepness * (i - start.x) : INT_MAX;
if (yi == INT_MAX) break;
std::cout << "(" << i << ", " << yi << ")";
if (yi != end.y) {
std::cout << " -> ";
}
}
std::cout << std::endl;
}
}
// 示例:绘制从(0,0)到(5,4),间隔为1的折线
std::vector<Point> complexLine = {{0, 0}, {5, 4}};
for (const auto& p : complexLine) {
drawLine(p, complexLine[(complexLine.size() + 1) % complexLine.size()], 1);
}
```
这个例子只处理了基本的折线情况,对于更复杂的折线形状(比如拐角或分支),你可能需要设计更复杂的逻辑来确定如何添加间隔。同时,这只是一个控制台输出的例子,如果你是在图形界面环境中,你需要使用相应的图形库(如SFML或OpenGL)来绘制实际图像。
阅读全文
相关推荐
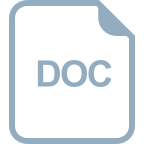
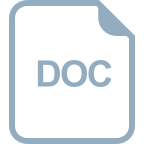
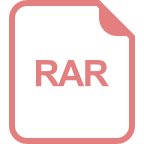
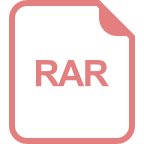
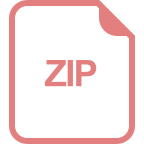
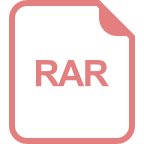
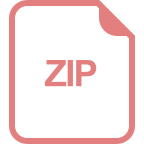
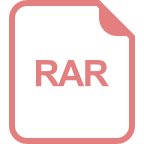
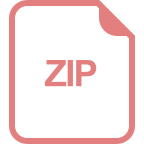
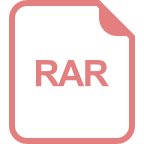