用Java代码写一个实时显示当前时间和天气和待办事项的程序
时间: 2024-10-08 19:20:54 浏览: 29
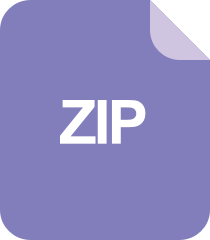
MyGroceryList:我已经构建了一个待办事项列表网络应用程序,但是我将其概括为一个杂货清单应用程序
在Java中创建一个实时显示当前时间、天气以及待办事项的程序,我们需要分别集成几个API来获取这些信息,并使用 Swing 或 JavaFX 等 GUI 框架来展示。这里是一个简化版的示例,我们将使用 JavaFX 来构建界面:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import java.time.LocalDateTime;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class TimeWeatherTodoApp extends Application {
private Label timeLabel, weatherLabel, todoList;
@Override
public void start(Stage primaryStage) {
// 设置窗口
primaryStage.setTitle("实时时间、天气和待办事项");
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 300);
// 获取并显示当前时间
timeLabel = createTimeLabel();
root.getChildren().add(timeLabel);
// 获取并显示天气信息(假设有一个返回JSON的天气API)
String weatherApiUrl = "https://api.weather.com/weather";
getWeatherInfo(weatherApiUrl, scene);
// 模拟待办事项列表
todoList = createTodoList();
root.getChildren().add(todoList);
primaryStage.setScene(scene);
primaryStage.show();
}
private Label createTimeLabel() {
Label label = new Label(LocalDateTime.now().format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")));
return label;
}
private void getWeatherInfo(String apiUrl, Scene scene) {
try {
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
if (conn.getResponseCode() == HttpURLConnection.HTTP_OK) {
String response = conn.getInputStream().toString();
JSONObject json = new JSONObject(response);
// 解析 JSON 并更新天气标签内容
// 这里只是一个示例,实际需要根据API文档解析数据
weatherLabel = new Label(json.getString("weatherDescription"));
scene.getChildren().add(weatherLabel);
}
} catch (Exception e) {
System.out.println("Failed to fetch weather info: " + e.getMessage());
}
}
private Label createTodoList() {
Label listLabel = new Label("待办事项:");
todoList = new Label("1. 购买食材\n" +
"2. 完成报告\n" +
"3. 锻炼身体"); // 假设这是硬编码的,实际应用应该从数据库或文件读取
return listLabel;
}
public static void main(String[] args) {
launch(args);
}
}
```
阅读全文
相关推荐
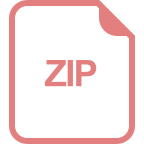
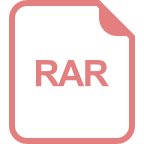
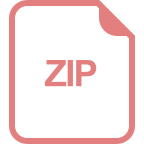
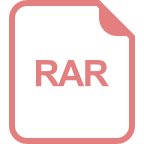
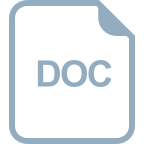
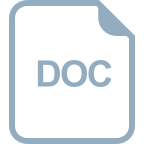
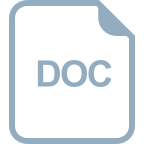
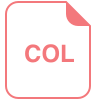
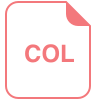
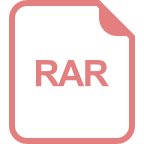
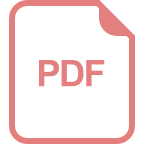
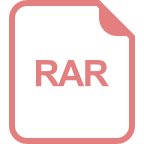
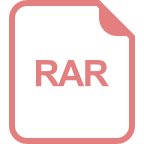