用c语言的方式求,在数组指定处,插入任意一个数据
时间: 2024-09-12 20:05:19 浏览: 37
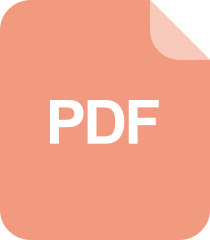
使用C语言实现vector动态数组的实例分享
在C语言中,你可以通过以下步骤在一个给定数组中插入一个元素:
1. 首先,你需要确定插入的位置,通常这需要遍历数组找到指定索引。如果索引小于0或大于数组长度减一,则表示错误的插入位置。
```c
#include <stdio.h>
int find_insertion_index(int array[], int size, int insert_value, int target_index) {
if (target_index < 0 || target_index > size - 1) {
printf("Invalid index.\n");
return -1; // 返回一个无效的位置或抛出异常
}
return target_index;
}
void insert_into_array(int array[], int size, int insert_value, int target_index) {
int new_size = size + 1; // 计算新数组的大小
int* newArray = realloc(array, sizeof(int) * new_size); // 动态分配新空间
if (newArray == NULL) { // 如果内存分配失败
perror("Memory allocation failed.");
exit(EXIT_FAILURE);
}
// 将原数组移动到新数组的相应位置,然后将新值插入
for (int i = 0; i <= target_index; ++i) {
newArray[i] = array[i];
}
newArray[target_index + 1] = insert_value;
// 释放旧数组的内存
free(array);
array = newArray;
size = new_size;
}
```
要使用这个函数,你可以像下面这样调用:
```c
int main() {
int my_array[] = {1, 2, 3, 4, 5};
int array_size = sizeof(my_array) / sizeof(my_array[0]);
int insert_value = 6;
int target_index = 3; // 想要插入的位置
int insertion_index = find_insertion_index(my_array, array_size, insert_value, target_index);
if (insertion_index != -1) {
insert_into_array(my_array, array_size, insert_value, insertion_index);
printf("Array after insertion: ");
for (int i = 0; i < array_size; i++) {
printf("%d ", my_array[i]);
}
}
return 0;
}
```
阅读全文
相关推荐
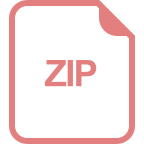














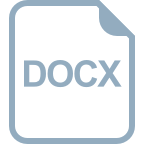
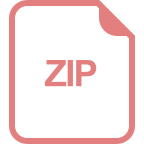