写一个程序小猿口算自动答题脚本
时间: 2024-10-13 09:00:58 浏览: 5
创建一个程序小猿口算自动答题脚本通常需要考虑以下几个步骤:
1. **环境准备**:
首先确定编程语言,比如Python,因为它的简洁性和丰富的库适合这种任务。安装相关的库如`requests`用于发送HTTP请求,`BeautifulSoup`解析网页内容。
2. **获取题目**:
访问提供口算题目的网站,如一些在线教育平台或小程序API。通过网络请求获取题目数据,并解析返回的数据,提取出需要计算的问题。
```python
import requests
from bs4 import BeautifulSoup
def fetch_problem():
url = "http://example.com/oracalc" # 替换为实际的题目API地址
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
problem = soup.find('div', class_='problem') # 类名假设题目在该标签内
if problem:
return problem.text # 提取并返回题目内容
else:
return None
```
3. **处理用户输入**:
创建一个函数接收用户的输入,可以使用内置的input()函数,然后进行验证和计算。
```python
def user_input(problem):
while True:
user_answer = input(f"{problem} = ")
try:
answer = int(user_answer)
break
except ValueError:
print("请输入数字结果!")
return answer
```
4. **提交答案**:
模拟用户点击提交按钮,通常这需要发送POST请求到服务器,并包含用户的答案。
```python
def submit_answer(problem, user_answer):
answer_data = {'question': problem, 'answer': user_answer}
headers = {'Content-Type': 'application/json'} # 如果网站需要特定的头信息
response = requests.post('http://example.com/submit', json=answer_data, headers=headers)
if response.status_code == 200:
print("答对了!")
else:
print("答案错误,请再试一次。")
```
5. **主循环和错误处理**:
将上述功能组合起来,在一个无限循环中运行,直到手动停止或者遇到网络问题等。
```python
while True:
problem = fetch_problem()
if not problem:
print("暂无新的题目,稍后再试。")
continue
user_answer = user_input(problem)
submit_answer(problem, user_answer)
```
相关推荐
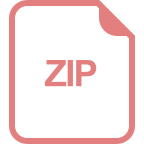
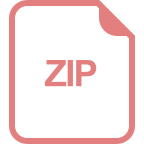
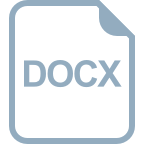





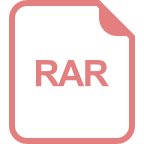
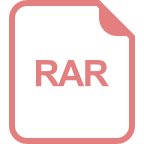
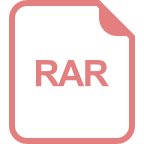
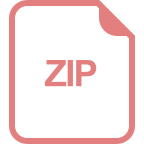
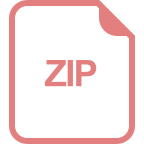
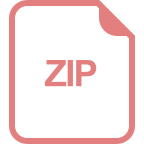

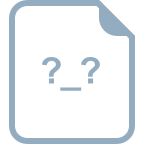
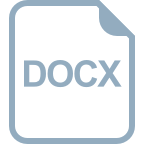