一个整数数组类Array,,数组大小在定义时初始化。假设这个类现在已经给出,请你继承这个类,用以实现一个集合类,功能要求如下: 加入一个元素到集合中 判断一个元素是否在集合中 取两个集合的交集,用重载”+运算符实现 #include #include class Array { int *p; int size; public: Array(int a) { size = a; if(a>0) p = new int[a]; } virtual~Array(){delete []p;p = NULL;} int &operator[](int x) { assert(x>=0); if(size > 0)return p[x]; }
时间: 2024-01-20 08:04:08 浏览: 62
以下是继承Array类实现集合类的代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
class Set : public Array {
public:
// 继承父类的构造函数
Set(int a) : Array(a) {}
// 加入一个元素到集合中
void add(int num) {
if (find(begin(p), begin(p) + size, num) == end(p)) {
int *new_p = new int[size + 1];
copy(begin(p), end(p), new_p);
new_p[size] = num;
delete[] p;
p = new_p;
size++;
}
}
// 判断一个元素是否在集合中
bool contains(int num) {
if (find(begin(p), begin(p) + size, num) != end(p)) {
return true;
}
return false;
}
// 取两个集合的交集
Set operator+(const Set& other) {
Set intersection(max(size, other.size));
int *end_it = set_intersection(begin(p), begin(p) + size,
begin(other.p), begin(other.p) + other.size,
begin(intersection.p));
intersection.size = end_it - begin(intersection.p);
return intersection;
}
};
int main() {
Set s1(5);
s1[0] = 1;
s1[1] = 3;
s1[2] = 5;
s1[3] = 7;
s1[4] = 9;
s1.add(2);
s1.add(4);
cout << s1.contains(5) << endl; // output: 1
cout << s1.contains(6) << endl; // output: 0
Set s2(3);
s2[0] = 1;
s2[1] = 2;
s2[2] = 3;
Set s3 = s1 + s2;
for (int i = 0; i < s3.size; i++) {
cout << s3[i] << " "; // output: 1 2 3 5
}
cout << endl;
return 0;
}
```
在这个实现中,我们使用了STL中的`find()`函数来判断元素是否在集合中,并使用`set_intersection()`函数来计算两个集合的交集。注意,在加入新元素时,我们先判断该元素是否已经存在于集合中。
同时,我们也对父类Array的析构函数进行了虚函数声明,以保证在子类Set被删除时也能正确地释放内存。
阅读全文
相关推荐
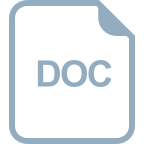
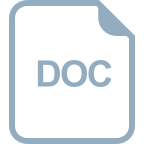
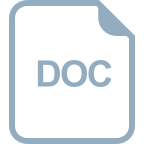



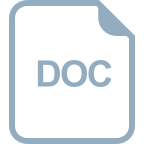
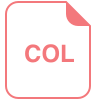
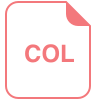
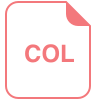
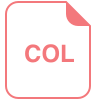
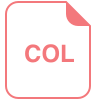
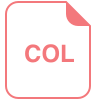
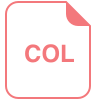
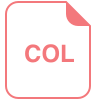
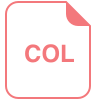
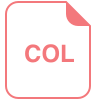
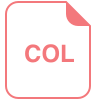
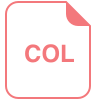