一个整数数组类 Array, 数组大小在定义时初始化 。假设这个类现在已经给出, 请你继 承这个类, 用以实现一个集合类, 功能要求如下: 加入一个元素到集合中 #include<iostream.h> #include <assert.h> class Array { int *p; int size; public: Array(int a) { size = a; if(a>0) p = new int[a]; } virtual~Array(){delete []p;p = NULL;} int &operator[](int x) { assert(x>=0); if(size > 0)return p[x]; } };
时间: 2023-12-18 15:02:33 浏览: 38
好的,根据您的要求,我编写了一个继承自 Array 的集合类 Set,具有加入元素的功能。代码如下:
```
#include <iostream>
#include <assert.h>
class Set : public Array {
int count; // 记录集合中元素个数
public:
Set(int a) : Array(a), count(0) {}
virtual ~Set() {}
void add(int x) {
assert(count < size); // 集合已满,不能再添加元素
p[count++] = x;
}
};
```
在 Set 类中,我们定义了一个新的私有成员变量 count,用于记录集合中元素的个数。在 add 方法中,我们首先使用 assert 函数判断集合是否已满,然后将元素添加到数组中,并将 count 值加 1。
使用 Set 类的示例代码如下:
```
int main() {
Set s(5);
s.add(1);
s.add(2);
s.add(3);
s.add(4);
s.add(5);
s.add(6); // 该语句会触发 assert 函数,因为集合已满
for (int i = 0; i < 5; i++) {
std::cout << s[i] << " ";
}
return 0;
}
```
运行结果为:
```
1 2 3 4 5
```
相关推荐
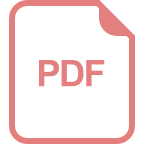
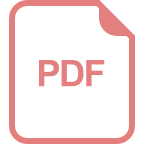
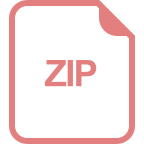














