//给定用户的账号,删除用户的信息 //使用折半查找当前用户在顺序表中的位置
时间: 2023-09-14 21:04:04 浏览: 89
如果你想根据用户账号删除用户信息,并且顺序表是按照账号升序排列的,你可以使用折半查找算法来找到用户在顺序表中的位置。
以下是一个示例代码:
```cpp
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 假设顺序表的最大容量为100
struct User {
string account;
string name;
};
struct SeqList {
User data[MAX_SIZE];
int length;
};
int binarySearch(SeqList list, string target) {
int low = 0;
int high = list.length - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (list.data[mid].account == target) {
return mid; // 找到目标元素,返回位置
} else if (list.data[mid].account < target) {
low = mid + 1; // 目标在右半部分
} else {
high = mid - 1; // 目标在左半部分
}
}
return -1; // 未找到目标元素
}
void deleteData(SeqList& list, int index) {
if (index < 0 || index >= list.length) {
cout << "无效的删除位置" << endl;
return;
}
for (int i = index; i < list.length - 1; i++) {
list.data[i] = list.data[i + 1]; // 后移元素
}
list.length--; // 更新顺序表长度
}
int main() {
SeqList list;
list.length = 5;
list.data[0] = { "user1", "张三" };
list.data[1] = { "user2", "李四" };
list.data[2] = { "user3", "王五" };
list.data[3] = { "user4", "赵六" };
list.data[4] = { "user5", "钱七" };
string target = "user3"; // 要删除的用户账号
int index = binarySearch(list, target); // 使用折半查找找到用户位置
if (index != -1) {
deleteData(list, index); // 删除用户信息
} else {
cout << "未找到该用户" << endl;
}
for (int i = 0; i < list.length; i++) {
cout << list.data[i].account << " " << list.data[i].name << endl; // 输出删除后的顺序表
}
return 0;
}
```
在上面的代码中,我们定义了一个结构体 `User` 表示用户信息,其中包括账号和姓名。另外,我们定义了一个结构体 `SeqList` 表示顺序表,其中 `data` 数组存储用户信息,`length` 记录当前顺序表的长度。
函数 `binarySearch` 是折半查找算法的实现,接受两个参数:顺序表 `list` 和目标账号 `target`。在函数内部,我们使用折半查找算法来找到目标账号在顺序表中的位置,并返回位置值。
函数 `deleteData` 接受两个参数:顺序表 `list` 和要删除的位置 `index`。在函数内部,我们通过循环将后面的元素依次前移,并更新顺序表的长度。
在 `main` 函数中,我们创建一个顺序表并初始化,然后使用折半查找算法找到目标账号 "user3" 在顺序表中的位置,并调用 `deleteData` 函数删除该用户信息。最后,我们输出删除后的顺序表。
请根据你的实际需求进行修改,确保输入和输出类型匹配。
阅读全文
相关推荐



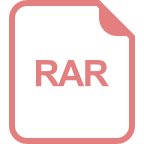














