用一个函数模板实现冒泡排序的功能,并在 main()函数中分别实现对 int 型数组和 double 型数组的排列。排序算法见教材 P252。 2. 用一个模板实现对栈的操作,栈的数据结构由链表实现。实现栈的功能: 进栈、出栈和清空的功能。并在 main()函数中实现对 int 型栈和 struct CHAPA 型栈 (定义如下)的基本操作。 //--------------------------------------------------------------------------------------------------- struct CHAPA { char *pName; bool Gender; int Age; struct CHAPA *next; };
时间: 2023-08-15 14:35:14 浏览: 112
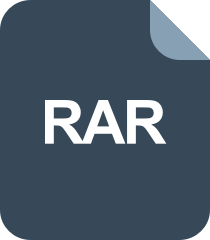
冒泡排序的模版
好的,我会根据您的要求进行回答。请注意,以下代码仅供参考。
1. 冒泡排序模板函数
```
template <typename T>
void bubbleSort(T arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
}
}
}
}
```
2. 链表栈模板类
```
template <typename T>
class LinkedListStack {
public:
LinkedListStack() {
head = nullptr;
count = 0;
}
~LinkedListStack() {
clear();
}
void push(T value) {
Node* node = new Node(value);
node->next = head;
head = node;
count++;
}
void pop() {
if (isEmpty()) {
throw runtime_error("Stack is empty!");
}
Node* node = head;
head = head->next;
delete node;
count--;
}
void clear() {
while (head != nullptr) {
Node* node = head;
head = head->next;
delete node;
}
count = 0;
}
bool isEmpty() {
return count == 0;
}
private:
struct Node {
T data;
Node* next;
Node(T value) : data(value), next(nullptr) {}
};
Node* head;
int count;
};
```
3. 在 main() 函数中使用模板函数和模板类
```
int main() {
// 冒泡排序
int arr1[] = { 3, 1, 4, 1, 5, 9, 2, 6, 5, 3 };
double arr2[] = { 3.14, 1.41, 5.92, 6.53, 5.89, 2.65 };
int n1 = sizeof(arr1) / sizeof(int);
int n2 = sizeof(arr2) / sizeof(double);
bubbleSort(arr1, n1);
bubbleSort(arr2, n2);
for (int i = 0; i < n1; i++) {
cout << arr1[i] << " ";
}
cout << endl;
for (int i = 0; i < n2; i++) {
cout << arr2[i] << " ";
}
cout << endl;
// 链表栈
LinkedListStack<int> intStack;
LinkedListStack<CHAPA*> chapaStack;
intStack.push(1);
intStack.push(2);
intStack.push(3);
while (!intStack.isEmpty()) {
cout << intStack.top() << " ";
intStack.pop();
}
cout << endl;
CHAPA chapa1 = { "Alice", false, 23, nullptr };
CHAPA chapa2 = { "Bob", true, 25, nullptr };
CHAPA chapa3 = { "Charlie", true, 22, nullptr };
chapaStack.push(&chapa1);
chapaStack.push(&chapa2);
chapaStack.push(&chapa3);
while (!chapaStack.isEmpty()) {
CHAPA* chapa = chapaStack.top();
cout << chapa->pName << " ";
chapaStack.pop();
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
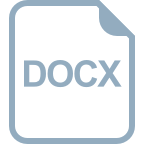
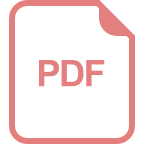















