linux使用c语言实现时间片轮转算法
时间: 2023-07-24 18:05:44 浏览: 101
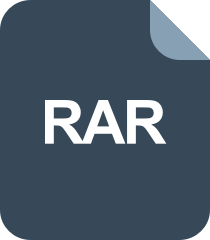
Time-slice-rotation-algorithm.rar_slice
下面是一个简单的使用 C 语言实现时间片轮转算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h>
#include <sys/time.h>
#define TIME_QUANTUM 1 // 时间片长度为 1 秒
#define MAX_PROCESSES 10 // 最大进程数
#define MAX_NAME_LEN 20 // 进程名最大长度
static int num_processes = 0; // 当前进程数
static int current_process = 0; // 当前运行的进程索引
static int time_left = 0; // 当前进程剩余时间
static int time_elapsed = 0; // 已经运行的时间
struct process {
char name[MAX_NAME_LEN];
int time_remaining;
int priority;
};
static struct process processes[MAX_PROCESSES];
// 定时器处理函数
void timer_handler(int signum) {
time_left--;
time_elapsed++;
// 当前进程时间用完,切换到下一个进程
if (time_left <= 0) {
current_process = (current_process + 1) % num_processes;
time_left = processes[current_process].time_remaining;
}
// 所有进程都已经运行完毕
if (time_elapsed >= MAX_PROCESSES * TIME_QUANTUM) {
printf("All processes have finished.\n");
exit(0);
}
}
// 注册定时器
void register_timer() {
struct sigaction sa;
struct itimerval timer;
// 安装定时器处理函数
sa.sa_handler = timer_handler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
sigaction(SIGALRM, &sa, NULL);
// 设置定时器
timer.it_value.tv_sec = TIME_QUANTUM;
timer.it_value.tv_usec = 0;
timer.it_interval.tv_sec = TIME_QUANTUM;
timer.it_interval.tv_usec = 0;
setitimer(ITIMER_REAL, &timer, NULL);
}
// 创建新进程
void create_process(char* name, int time_remaining, int priority) {
if (num_processes >= MAX_PROCESSES) {
printf("Too many processes.\n");
exit(1);
}
struct process p;
strncpy(p.name, name, MAX_NAME_LEN);
p.time_remaining = time_remaining;
p.priority = priority;
processes[num_processes++] = p;
}
int main() {
// 创建一些进程
create_process("process1", 3, 1);
create_process("process2", 5, 2);
create_process("process3", 2, 3);
register_timer();
// 执行进程
while (1) {
printf("[%d] Running process %s...\n", time_elapsed, processes[current_process].name);
sleep(1);
}
return 0;
}
```
在这个示例代码中,我们使用了 `setitimer` 函数来注册定时器,并且在定时器处理函数中实现了时间片轮转算法的核心逻辑。在 `create_process` 函数中,我们可以创建新的进程,并将它们添加到进程列表中。在 `main` 函数中,我们不断地执行当前进程,并在进程时间用完时切换到下一个进程。当所有进程都已经运行完毕时,程序将退出。
需要注意的是,这个示例代码非常简单,只是一个基本的框架,实际使用中还需要根据具体情况进行一些调整和优化。
阅读全文
相关推荐
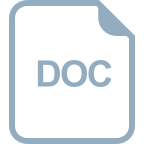
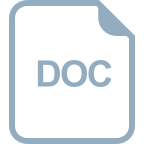














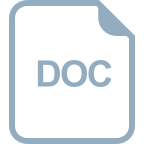
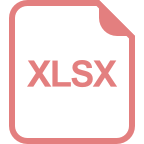
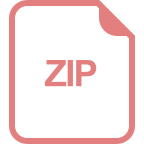