基于C语言的时间片轮转算法的模拟
时间: 2023-09-08 15:09:52 浏览: 40
好的,关于时间片轮转算法的模拟,我们可以考虑以下步骤:
1. 定义进程结构体
为了方便模拟,我们可以定义一个进程结构体,包含进程的ID、优先级、状态、运行时间、剩余时间等属性。
```
struct process {
int id; // 进程ID
int priority; // 进程优先级
int state; // 进程状态,0表示就绪,1表示运行,2表示完成
int run_time; // 进程已运行时间
int remain_time; // 进程剩余运行时间
};
```
2. 定义进程队列
为了实现时间片轮转算法,我们需要定义一个进程队列,用于存储所有就绪状态的进程。可以使用链表或数组来实现。
```
struct node {
struct process p;
struct node *next;
};
struct node *head = NULL; // 队列头指针
struct node *tail = NULL; // 队列尾指针
```
3. 添加进程到队列中
当一个进程创建时,它会被加入到就绪队列中。我们可以定义一个函数来实现这个功能。
```
void addProcess(int id, int priority, int run_time) {
struct process p;
p.id = id;
p.priority = priority;
p.state = 0;
p.run_time = 0;
p.remain_time = run_time;
struct node *newNode = (struct node*)malloc(sizeof(struct node));
newNode->p = p;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
```
4. 模拟时间片轮转算法
在时间片轮转算法中,每个进程只能运行一定时间,然后就被停止,让其他进程运行。我们可以通过循环来模拟这个过程。
```
void run() {
int time_slice = 2; // 时间片长度为2
struct node *current = head; // 当前运行的进程
while (head != NULL) {
// 遍历队列中的所有进程
struct node *p = head;
while (p != NULL) {
if (p->p.state == 0) {
// 进程处于就绪状态,将其加入到队列末尾
p->p.priority += 1; // 优先级加1
tail->next = p;
tail = p;
}
p = p->next;
}
// 运行当前进程
printf("Running process %d\n", current->p.id);
current->p.state = 1; // 进程状态改为运行
current->p.run_time += time_slice;
current->p.remain_time -= time_slice;
if (current->p.remain_time <= 0) {
// 进程已完成
printf("Process %d has finished.\n", current->p.id);
current->p.state = 2; // 进程状态改为完成
} else {
// 进程未完成,将其加入到队列末尾
current->p.priority -= 1; // 优先级减1
tail->next = current;
tail = current;
}
// 查找下一个可运行的进程
struct node *p = current->next;
while (p != current) {
if (p->p.state == 0) {
current = p;
break;
}
p = p->next;
}
}
}
```
以上就是基于C语言的时间片轮转算法的模拟的主要步骤,需要注意的是,本算法的时间片长度和进程的优先级可以根据实际需要进行调整。
相关推荐
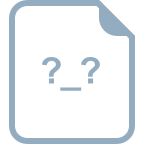














