【示例11】已知一颗二叉树的先序序列为EBADCFHG,其中序序列为 ABCDEFGH,还原二叉树过程
时间: 2024-01-23 10:11:20 浏览: 27
这道题可以通过递归的方法来还原二叉树,具体过程如下:
1. 先找到先序序列的第一个元素,即根节点,这里是E。
2. 在中序序列中找到根节点的位置,根节点前面的为左子树的中序序列,后面的为右子树的中序序列,这里是 ABCD 和 FGH。
3. 根据左子树的中序序列和先序序列,递归还原左子树。
4. 根据右子树的中序序列和先序序列,递归还原右子树。
5. 将左子树和右子树连接到根节点上。
具体实现过程如下Python代码所示:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def rebuild_tree(preorder, inorder):
if not preorder or not inorder:
return None
# 先序序列的第一个元素即为根节点
root = Node(preorder[0])
# 在中序序列中找到根节点的位置
index = inorder.index(root.val)
# 递归还原左子树
root.left = rebuild_tree(preorder[1:index+1], inorder[:index])
# 递归还原右子树
root.right = rebuild_tree(preorder[index+1:], inorder[index+1:])
return root
preorder = 'EBADCFHG'
inorder = 'ABCDEFGH'
root = rebuild_tree(preorder, inorder)
```
最后得到的root即为还原出来的二叉树的根节点。
相关推荐
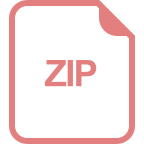
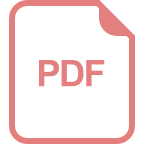
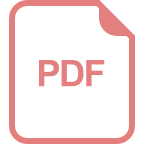














