写一个基于A*算法的voronoi图路径规划
时间: 2023-07-20 08:09:00 浏览: 53
首先,我们需要了解什么是A*算法和Voronoi图。
A*算法是一种启发式搜索算法,用于寻找最短路径。它利用启发式函数来估计从当前节点到目标节点的最短距离,并通过优先级队列来选择下一个要探索的节点。
Voronoi图是由一组离散点形成的图,其中每个点都是以该点为中心的最近邻点之间的垂直平分线。Voronoi图可用于路径规划,因为它可以将空间分割成区域,使得每个区域内的点都更接近于该区域内的离散点。
现在,我们可以开始编写基于A*算法的Voronoi图路径规划。
1. 首先,我们需要确定起始点和目标点。这些点可以是随机选择的,也可以是根据特定的规划问题选择的。
2. 接下来,我们需要生成Voronoi图。我们可以使用Python的scipy库来实现这一点。具体来说,我们可以使用scipy.spatial.Voronoi函数来生成Voronoi图。该函数将返回一个包含Voronoi图中的顶点、边和面的对象。
3. 接下来,我们需要计算起始点和目标点在Voronoi图上的位置。这可以通过找到最接近的Voronoi图顶点来实现。
4. 然后,我们可以使用A*算法来查找起始点到目标点的最短路径。我们可以使用Python的heapq模块来实现优先级队列。具体来说,我们可以将所有未探索的节点添加到队列中,并按其到目标点的估计距离排序。然后,我们可以选择距离目标点最近的节点,并从队列中删除它。接下来,我们可以将该节点标记为已探索,并检查其相邻节点。对于每个相邻节点,我们可以计算从起始点到该节点的实际距离,并通过计算从该节点到目标点的估计距离来计算该节点的总成本。如果该节点尚未被探索过或者其总成本比之前找到的路径更短,则将其添加到优先级队列中。
5. 最后,我们可以通过连接所有经过的Voronoi图顶点来生成路径。
下面是一个基于A*算法的Voronoi图路径规划的Python代码示例:
```python
import heapq
import numpy as np
from scipy.spatial import Voronoi
def generate_voronoi(points):
vor = Voronoi(points)
return vor
def get_closest_voronoi_vertex(vor, point):
distances = np.linalg.norm(vor.vertices - point, axis=1)
closest_vertex_index = np.argmin(distances)
return vor.vertices[closest_vertex_index]
def a_star_search(start, goal, vor):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
current = heapq.heappop(frontier)[1]
if current == goal:
break
for neighbor in get_neighbors(vor, current):
new_cost = cost_so_far[current] + distance(current, neighbor)
if neighbor not in cost_so_far or new_cost < cost_so_far[neighbor]:
cost_so_far[neighbor] = new_cost
priority = new_cost + heuristic(goal, neighbor)
heapq.heappush(frontier, (priority, neighbor))
came_from[neighbor] = current
path = [goal]
current = goal
while current != start:
current = came_from[current]
path.append(current)
path.reverse()
return path
def get_neighbors(vor, point):
for simplex in vor.ridge_vertices:
if -1 not in simplex and point in simplex:
yield vor.vertices[simplex[simplex.index(point) ^ 1]]
def distance(p1, p2):
return np.linalg.norm(np.array(p1) - np.array(p2))
def heuristic(p1, p2):
return distance(p1, p2)
if __name__ == '__main__':
points = np.random.rand(50, 2)
start = (0.1, 0.1)
goal = (0.9, 0.9)
vor = generate_voronoi(points)
start_vor = get_closest_voronoi_vertex(vor, start)
goal_vor = get_closest_voronoi_vertex(vor, goal)
path = a_star_search(start_vor, goal_vor, vor)
print(path)
```
在此示例中,我们首先生成了50个随机点,并使用这些点来生成Voronoi图。然后,我们选择起始点和目标点,并找到它们在Voronoi图上的最接近的顶点。接下来,我们使用A*算法来查找起始点到目标点的最短路径。最后,我们通过连接路径上的顶点来构建路径。
相关推荐
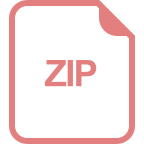
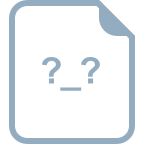
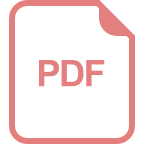














