python+tkinter实现读取图像视频+彩色图像转换为灰度图+prewitt实现车道线边缘检测+生成 Mask 掩模+直线检测+绘制车道线
时间: 2023-08-09 07:10:02 浏览: 144
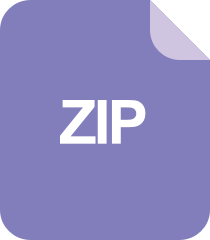
基于python+opencv制作的工业图像检测处理工具项目代码.zip
好的,下面是完整的代码实现:
```python
import cv2
import numpy as np
import tkinter as tk
from PIL import Image, ImageTk
# 读取视频
cap = cv2.VideoCapture('video.mp4')
# 创建 Tkinter 窗口
root = tk.Tk()
# 创建 Canvas 组件
canvas = tk.Canvas(root, width=cap.get(3), height=cap.get(4))
canvas.pack()
while True:
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 将彩色图像转换为灰度图
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 定义 Prewitt 模板
prewitt_x = np.array([[-1, 0, 1], [-1, 0, 1], [-1, 0, 1]])
prewitt_y = np.array([[-1, -1, -1], [0, 0, 0], [1, 1, 1]])
# 对灰度图像进行卷积操作
grad_x = cv2.filter2D(gray, -1, prewitt_x)
grad_y = cv2.filter2D(gray, -1, prewitt_y)
# 计算梯度幅值
grad = np.sqrt(grad_x ** 2 + grad_y ** 2)
# 将梯度幅值转换为 0-255 范围内的灰度值
grad = ((grad - grad.min()) / (grad.max() - grad.min()) * 255).astype(np.uint8)
# 生成多边形掩模
h, w = grad.shape
vertices = np.array([[(0, h), (w // 2, h // 2), (w, h)]], dtype=np.int32)
mask = np.zeros_like(grad)
cv2.fillPoly(mask, vertices, 255)
masked_grad = cv2.bitwise_and(grad, mask)
# 进行直线检测
lines = cv2.HoughLinesP(masked_grad, rho=1, theta=np.pi/180, threshold=50, minLineLength=50, maxLineGap=5)
# 绘制车道线
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(frame, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 在 Canvas 上显示帧
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = Image.fromarray(img)
img_tk = ImageTk.PhotoImage(img)
canvas.create_image(0, 0, anchor=tk.NW, image=img_tk)
canvas.update()
# 关闭视频
cap.release()
```
代码中的注释比较详细,主要思路是:
1. 使用 OpenCV 库读取视频,使用 Tkinter 库创建 GUI 界面,并在 Canvas 组件上显示视频帧。
2. 使用 cv2.cvtColor() 函数将彩色图像转换为灰度图。
3. 定义 Prewitt 算子模板,使用 cv2.filter2D() 函数对灰度图像进行卷积操作,得到图像的梯度幅值。
4. 使用 cv2.fillPoly() 函数生成多边形掩模,将不需要检测的区域遮盖掉,得到需要检测的区域。
5. 使用 cv2.HoughLinesP() 函数进行直线检测,得到图像中的直线。
6. 使用 cv2.line() 函数在图像上绘制车道线。
7. 使用 Image.fromarray() 函数将图像数组转换为 PIL.Image 对象,然后使用 ImageTk.PhotoImage() 函数将 PIL.Image 对象转换为 Tkinter 可以显示的图像格式。
8. 使用 Canvas.create_image() 函数在 Canvas 上显示图像,使用 Canvas.update() 函数刷新界面。
希望能对你有所帮助!
阅读全文
相关推荐
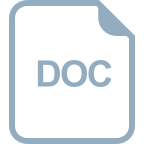
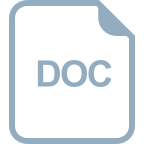

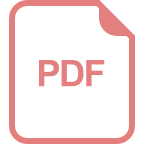
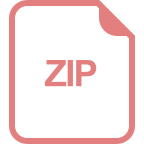
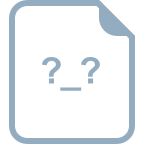
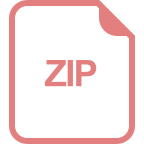
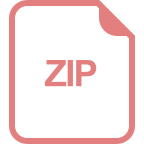
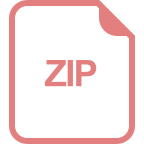
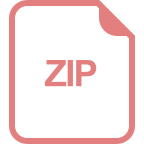
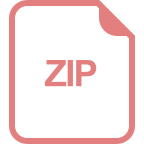
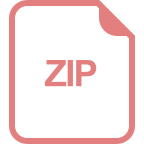
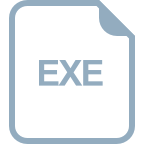
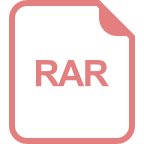
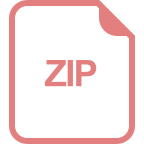
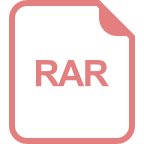