python+tkinter+prewitt实现车道线边缘检测
时间: 2024-01-03 12:05:38 浏览: 27
首先,需要导入相关的库文件:
```python
import tkinter as tk
from tkinter import filedialog
from PIL import ImageTk, Image
import numpy as np
import cv2
```
然后,创建一个窗口,并且定义一个函数用于选择图片:
```python
root = tk.Tk()
root.title("Lane Detection")
def select_image():
global img_pil, img_tk, img_gray
path = filedialog.askopenfilename()
img_pil = Image.open(path)
img_tk = ImageTk.PhotoImage(img_pil)
img_gray = np.asarray(img_pil.convert('L'))
canvas.itemconfig(image_on_canvas, image=img_tk)
```
接下来,创建一个画布和一个按钮,用于选择图片:
```python
canvas = tk.Canvas(root, width=500, height=500)
canvas.pack(side=tk.LEFT)
button = tk.Button(root, text="Select Image", command=select_image)
button.pack(side=tk.RIGHT)
```
然后,定义一个函数用于实现车道线边缘检测:
```python
def edge_detection():
global img_pil, img_tk, img_gray
kernel = np.array([[-1,0,1],[-1,0,1],[-1,0,1]])
img_edges = cv2.filter2D(img_gray, -1, kernel)
img_edges = cv2.convertScaleAbs(img_edges)
img_pil = Image.fromarray(img_edges)
img_tk = ImageTk.PhotoImage(img_pil)
canvas.itemconfig(image_on_canvas, image=img_tk)
```
最后,在画布上显示图片:
```python
img_tk = ImageTk.PhotoImage(Image.new('RGB', (500, 500), (255, 255, 255)))
image_on_canvas = canvas.create_image(0, 0, image=img_tk, anchor=tk.NW)
```
完整代码如下:
```python
import tkinter as tk
from tkinter import filedialog
from PIL import ImageTk, Image
import numpy as np
import cv2
root = tk.Tk()
root.title("Lane Detection")
def select_image():
global img_pil, img_tk, img_gray
path = filedialog.askopenfilename()
img_pil = Image.open(path)
img_tk = ImageTk.PhotoImage(img_pil)
img_gray = np.asarray(img_pil.convert('L'))
canvas.itemconfig(image_on_canvas, image=img_tk)
def edge_detection():
global img_pil, img_tk, img_gray
kernel = np.array([[-1,0,1],[-1,0,1],[-1,0,1]])
img_edges = cv2.filter2D(img_gray, -1, kernel)
img_edges = cv2.convertScaleAbs(img_edges)
img_pil = Image.fromarray(img_edges)
img_tk = ImageTk.PhotoImage(img_pil)
canvas.itemconfig(image_on_canvas, image=img_tk)
canvas = tk.Canvas(root, width=500, height=500)
canvas.pack(side=tk.LEFT)
button = tk.Button(root, text="Select Image", command=select_image)
button.pack(side=tk.RIGHT)
img_tk = ImageTk.PhotoImage(Image.new('RGB', (500, 500), (255, 255, 255)))
image_on_canvas = canvas.create_image(0, 0, image=img_tk, anchor=tk.NW)
button_edge_detection = tk.Button(root, text="Edge Detection", command=edge_detection)
button_edge_detection.pack(side=tk.BOTTOM)
root.mainloop()
```
相关推荐
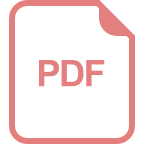
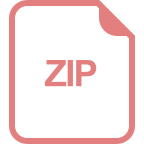
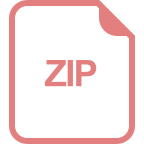
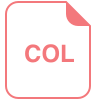
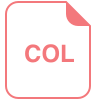
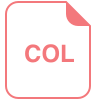
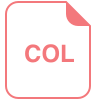
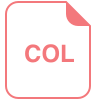









