opencv人脸识别c++代码yolo模型
时间: 2023-07-24 19:56:07 浏览: 42
以下是使用OpenCV和YOLO模型进行人脸识别的C++代码示例:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/dnn.hpp>
using namespace cv;
using namespace dnn;
int main()
{
// 加载YOLO模型
String modelConfiguration = "yolov3.cfg";
String modelWeights = "yolov3.weights";
Net net = readNetFromDarknet(modelConfiguration, modelWeights);
// 加载类别标签
std::vector<String> classNames;
String classesFile = "coco.names";
std::ifstream ifs(classesFile.c_str());
std::string line;
while (std::getline(ifs, line))
{
classNames.push_back(line);
}
// 加载图像
Mat image = imread("input.jpg");
// 创建blob
Mat blob;
double scalefactor = 1 / 255.0;
Size size = Size(416, 416);
Scalar mean = Scalar(0, 0, 0);
bool swapRB = true;
bool crop = false;
blobFromImage(image, blob, scalefactor, size, mean, swapRB, crop);
// 设置输入层
net.setInput(blob);
// 执行前向传播
std::vector<Mat> outs;
std::vector<String> outNames = net.getUnconnectedOutLayersNames();
net.forward(outs, outNames);
// 解析输出层
std::vector<int> classIds;
std::vector<float> confidences;
std::vector<Rect> boxes;
for (size_t i = 0; i < outs.size(); ++i)
{
// 获取检测结果
float* data = (float*)outs[i].data;
for (int j = 0; j < outs[i].rows; ++j, data += outs[i].cols)
{
Mat scores = outs[i].row(j).colRange(5, outs[i].cols);
Point classIdPoint;
double confidence;
minMaxLoc(scores, 0, &confidence, 0, &classIdPoint);
if (confidence > 0.5)
{
int centerX = (int)(data[0] * image.cols);
int centerY = (int)(data[1] * image.rows);
int width = (int)(data[2] * image.cols);
int height = (int)(data[3] * image.rows);
int left = centerX - width / 2;
int top = centerY - height / 2;
classIds.push_back(classIdPoint.x);
confidences.push_back((float)confidence);
boxes.push_back(Rect(left, top, width, height));
}
}
}
// 非最大抑制
std::vector<int> indices;
NMSBoxes(boxes, confidences, 0.5, 0.4, indices);
// 绘制检测结果
for (size_t i = 0; i < indices.size(); ++i)
{
int idx = indices[i];
Rect box = boxes[idx];
// 绘制边界框
rectangle(image, box, Scalar(0, 0, 255), 2);
// 绘制类别标签和置信度
String label = classNames[classIds[idx]] + ": " + std::to_string(confidences[idx]);
int baseLine;
Size labelSize = getTextSize(label, FONT_HERSHEY_SIMPLEX, 0.5, 1, &baseLine);
int top = std::max(box.y, labelSize.height);
rectangle(image, Point(box.x, top - labelSize.height),
Point(box.x + labelSize.width, top + baseLine), Scalar(255, 255, 255), FILLED);
putText(image, label, Point(box.x, top), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 0, 0));
}
// 显示结果
imshow("Face Detection", image);
waitKey(0);
return 0;
}
```
请确保正确配置YOLO模型文件(`.cfg`和`.weights`文件)以及类别标签文件(`.names`文件)。此代码将加载一张名为`input.jpg`的图像,并在图像中检测和标记人脸。
相关推荐
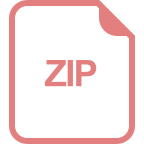
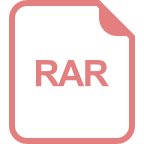
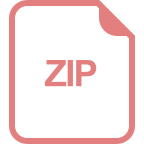














