用Runnable接口编写class SimpleThread extends Thread { public SimpleThread(String str) { super( str ); } public void run() { for (int i = 0; i < 10; i++) { System.out.println( i + "" + getName() ); try { sleep( (int) (Math.random() * 1000) ); } catch (InterruptedException e) { } } System.out.println( "DONE!" + getName() ); } }public class TwoThreadsTest { public static void main(String args[]) { new SimpleThread( "First" ).start(); new SimpleThread( "Second" ).start(); } }
时间: 2024-03-25 15:35:30 浏览: 60
可以使用 Runnable 接口来实现类似的功能。修改后的代码如下:
```
class SimpleRunnable implements Runnable {
private String name;
public SimpleRunnable(String name) {
this.name = name;
}
public void run() {
for (int i = 0; i < 10; i++) {
System.out.println(i + "" + name);
try {
Thread.sleep((int) (Math.random() * 1000));
} catch (InterruptedException e) {
}
}
System.out.println("DONE!" + name);
}
}
public class TwoThreadsTest {
public static void main(String args[]) {
new Thread(new SimpleRunnable("First")).start();
new Thread(new SimpleRunnable("Second")).start();
}
}
```
这里,定义了一个实现了 Runnable 接口的 SimpleRunnable 类,其中包含一个 name 属性,用于存储线程的名称。在 run() 方法中,执行了和之前一样的循环和输出操作。最后,在 main() 方法中,创建了两个线程,并将 SimpleRunnable 对象作为参数传递给 Thread 构造函数。然后,通过 start() 方法启动线程。运行结果和之前的例子类似。
阅读全文
相关推荐
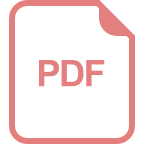
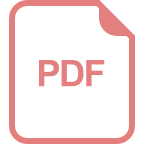
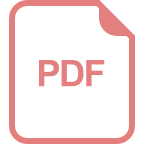

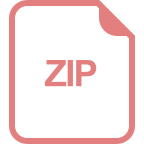
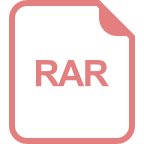
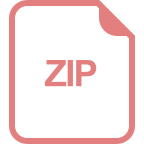
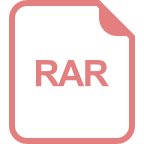
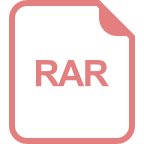
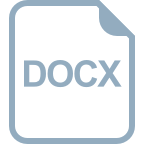
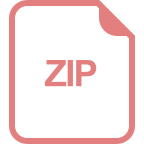
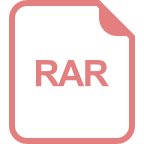
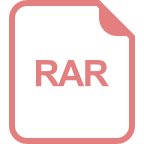
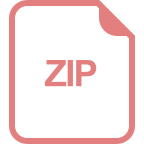
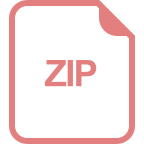