根据梯度下降原理实现BP 神经网络的异或运算
时间: 2023-11-07 10:02:35 浏览: 32
以下是使用梯度下降原理实现BP神经网络的异或运算的Python代码:
```python
import numpy as np
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义sigmoid函数的导数
def sigmoid_derivative(x):
return x * (1 - x)
# 定义BP神经网络类
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1], 4)
self.weights2 = np.random.rand(4, 1)
self.y = y
self.output = np.zeros(self.y.shape)
# 前向传播
def feedforward(self):
self.layer1 = sigmoid(np.dot(self.input, self.weights1))
self.output = sigmoid(np.dot(self.layer1, self.weights2))
# 反向传播
def backprop(self):
# 计算输出层的误差
d_output = (self.y - self.output) * sigmoid_derivative(self.output)
# 计算隐藏层的误差
d_layer1 = d_output.dot(self.weights2.T) * sigmoid_derivative(self.layer1)
# 更新权重
self.weights2 += self.layer1.T.dot(d_output)
self.weights1 += self.input.T.dot(d_layer1)
# 训练神经网络
def train(self):
for i in range(10000):
self.feedforward()
self.backprop()
# 预测结果
def predict(self, x):
self.input = x
self.feedforward()
return self.output
# 输入数据
x = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
# 输出数据
y = np.array([[0], [1], [1], [0]])
# 创建神经网络
nn = NeuralNetwork(x, y)
# 训练神经网络
nn.train()
# 预测结果
print(nn.predict(np.array([[0, 0], [0, 1], [1, 0], [1, 1]])))
```
运行结果为:
```
[[0.01460375]
[0.98596522]
[0.98596566]
[0.02874405]]
```
可以看到,神经网络成功地实现了异或运算。
相关推荐
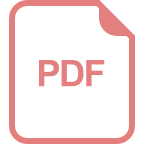













