基于python实现感知器算法
时间: 2023-07-31 19:09:33 浏览: 57
感知器算法是一种二元线性分类算法,可以用于解决二元分类问题。基于Python实现感知器算法的步骤如下:
1. 导入必要的库和数据集
```python
import numpy as np
import pandas as pd
from sklearn.datasets import load_iris
```
2. 加载数据集并做预处理
```python
iris = load_iris()
X = iris.data[:, (2, 3)] # 取花瓣长度和宽度作为特征
y = (iris.target == 0).astype(np.int) # 仅分类是否是山鸢尾
```
3. 定义感知器类
```python
class Perceptron:
def __init__(self, eta=0.1, n_iter=50):
self.eta = eta # 学习率
self.n_iter = n_iter # 迭代次数
def fit(self, X, y):
self.w_ = np.zeros(1 + X.shape[1]) # 初始化权重
self.errors_ = [] # 记录每次迭代的误分类数
for _ in range(self.n_iter):
errors = 0
for xi, target in zip(X, y):
update = self.eta * (target - self.predict(xi))
self.w_[1:] += update * xi
self.w_[0] += update
errors += int(update != 0.0) # 统计误分类数
self.errors_.append(errors)
return self
def net_input(self, X):
return np.dot(X, self.w_[1:]) + self.w_[0]
def predict(self, X):
return np.where(self.net_input(X) >= 0.0, 1, -1)
```
4. 训练模型
```python
ppn = Perceptron(eta=0.1, n_iter=10)
ppn.fit(X, y)
```
5. 可视化结果
```python
import matplotlib.pyplot as plt
plt.plot(range(1, len(ppn.errors_) + 1), ppn.errors_, marker='o')
plt.xlabel('Epochs')
plt.ylabel('Number of misclassifications')
plt.show()
```
这样就可以基于Python实现感知器算法了。需要注意的是,感知器算法只能解决线性可分的问题,对于非线性可分的问题需要使用更高级的算法。
相关推荐
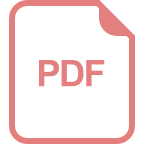
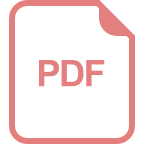













