写一个完整的图片上传的例子
时间: 2024-04-13 14:30:14 浏览: 13
下面是一个使用Java编写的完整图片上传的例子,基于Spring Boot框架和Apache Commons FileUpload库:
1. 创建一个Controller类,用于接收上传请求并保存上传的图片文件。
```java
import org.apache.commons.io.FilenameUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@RestController
public class ImageUploadController {
@Value("${upload.directory}")
private String uploadDirectory;
@PostMapping("/upload")
public ResponseEntity<String> uploadImage(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return new ResponseEntity<>("No file uploaded", HttpStatus.BAD_REQUEST);
}
try {
String originalFilename = StringUtils.cleanPath(file.getOriginalFilename());
String extension = FilenameUtils.getExtension(originalFilename);
String newFileName = System.currentTimeMillis() + "." + extension;
File destFile = new File(uploadDirectory + newFileName);
file.transferTo(destFile);
// 这里可以根据需求对上传成功后的逻辑进行处理
return new ResponseEntity<>("File uploaded successfully", HttpStatus.OK);
} catch (IOException e) {
e.printStackTrace();
return new ResponseEntity<>("Failed to upload file", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
2. 在application.properties文件中配置上传文件的存储目录。
```properties
upload.directory=/path/to/upload/directory/
```
3. 在Spring Boot的启动类上添加注解@EnableWebMvc。
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
@SpringBootApplication
@EnableWebMvc
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
以上示例中,通过@RequestParam注解接收前端传来的文件参数,然后使用MultipartFile类型来保存上传的文件。在保存文件时,使用了Apache Commons FileUpload库中的transferTo方法将文件保存到指定的目录中。
请注意将`/path/to/upload/directory/`替换为你希望保存上传文件的实际目录。
这个例子是基于Spring Boot和Apache Commons FileUpload库实现的,你也可以使用其他框架或库来完成图片上传,具体实现可能会有所不同。
相关推荐
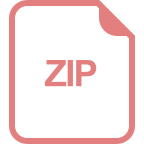














