用循环体输入生成二叉树,输入quit停止生成二叉树
时间: 2023-12-03 08:43:58 浏览: 67
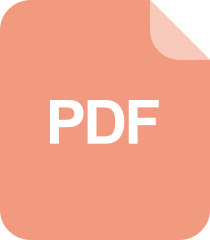
JavaScript数据结构与算法之二叉树插入节点、生成二叉树示例
好的,这里给出一个用循环体输入生成二叉树的示例代码:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def build_tree():
# 根节点
root_val = input("Enter the root value (quit to stop): ")
if root_val.lower() == "quit":
return None
root = TreeNode(int(root_val))
# 循环体输入左右子树
queue = [root]
while queue:
node = queue.pop(0)
# 输入左子树
left_val = input(f"Enter the left child of {node.val} (quit to stop): ")
if left_val.lower() != "quit":
left = TreeNode(int(left_val))
node.left = left
queue.append(left)
# 输入右子树
right_val = input(f"Enter the right child of {node.val} (quit to stop): ")
if right_val.lower() != "quit":
right = TreeNode(int(right_val))
node.right = right
queue.append(right)
return root
```
使用方法:
```python
root = build_tree()
```
示例输入:
```
Enter the root value (quit to stop): 1
Enter the left child of 1 (quit to stop): 2
Enter the right child of 1 (quit to stop): 3
Enter the left child of 2 (quit to stop): 4
Enter the right child of 2 (quit to stop): quit
Enter the left child of 3 (quit to stop): quit
Enter the right child of 3 (quit to stop): 5
Enter the left child of 4 (quit to stop): quit
Enter the right child of 4 (quit to stop): quit
Enter the left child of 5 (quit to stop): quit
Enter the right child of 5 (quit to stop): quit
```
生成的二叉树:
```
1
/ \
2 3
/ /
4 5
```
阅读全文
相关推荐
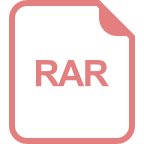
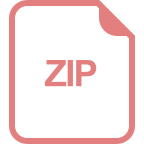















