能举例一个生产者模式和消费者模式的多线程代码吗
时间: 2023-10-08 16:08:42 浏览: 98
当然可以,以下是一个简单的生产者消费者模式的多线程代码实现:
```python
import threading
import time
# 定义全局变量,用于存放生产者生产的产品
products = []
# 定义一个互斥锁,防止多个线程同时访问 products 变量
lock = threading.Lock()
# 定义生产者线程类
class Producer(threading.Thread):
def run(self):
global products
while True:
if lock.acquire(): # 获取互斥锁
if len(products) < 10: # 如果 products 列表长度小于 10,说明可以继续生产
products.append("Product")
print("Producer produced a product. Now there are %d products in the list." % len(products))
lock.release() # 释放互斥锁
else:
print("Producer is waiting for consumer to consume the products.")
lock.release() # 释放互斥锁
time.sleep(1) # 等待 1 秒钟
# 定义消费者线程类
class Consumer(threading.Thread):
def run(self):
global products
while True:
if lock.acquire(): # 获取互斥锁
if len(products) > 0: # 如果 products 列表长度大于 0,说明可以继续消费
product = products.pop()
print("Consumer consumed a product. Now there are %d products in the list." % len(products))
lock.release() # 释放互斥锁
else:
print("Consumer is waiting for producer to produce the products.")
lock.release() # 释放互斥锁
time.sleep(1) # 等待 1 秒钟
# 创建一个生产者线程对象和一个消费者线程对象
producer_thread = Producer()
consumer_thread = Consumer()
# 启动生产者线程和消费者线程
producer_thread.start()
consumer_thread.start()
# 等待两个线程结束
producer_thread.join()
consumer_thread.join()
```
在这个例子中,生产者线程会不断地生产产品,直到产品数量达到上限(这里设置为 10),然后等待消费者线程消费产品。消费者线程会不断地消费产品,直到产品数量为 0,然后等待生产者线程生产产品。在生产者线程和消费者线程访问 products 变量时,会先获取一个互斥锁,以保证同一时间只有一个线程能够访问这个变量,从而避免出现竞争状态。
阅读全文
相关推荐
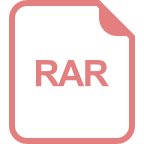
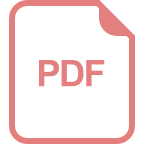
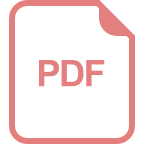
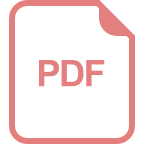
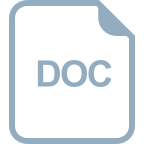
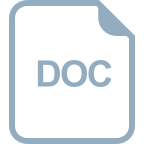
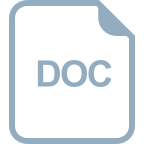
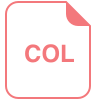
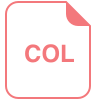
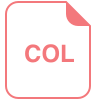
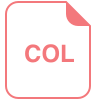
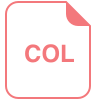
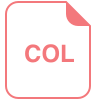
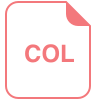
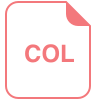
