编程实现打印出长度为M的unsigned int数组NUM中bit0~bit7最大的2个数。
时间: 2024-03-01 19:51:03 浏览: 41
可以使用桶排序的思想,先对数组中每个数的bit0~bit7进行计数排序,得到一个长度为256的桶数组count。然后从桶数组count的末尾向前遍历,找到前两个非零元素的下标,即为所求的最大的两个数。
以下是示例代码实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void findMaxTwo(unsigned int* NUM, int M) {
vector<int> count(256, 0);
for (int i = 0; i < M; ++i) {
++count[NUM[i] & 0xff]; // 对bit0~bit7进行计数排序
}
int max1 = 255, max2 = 255; // 初始化最大两个数的下标为255
for (int i = 255; i >= 0; --i) {
if (count[i] > 0) {
if (max1 == 255) {
max1 = i;
} else if (max2 == 255) {
max2 = i;
break;
}
}
}
cout << "The two maximum numbers are: " << max1 << ", " << max2 << endl;
}
int main() {
unsigned int NUM[] = {123, 456, 789, 321, 654, 987};
int M = sizeof(NUM) / sizeof(NUM[0]);
findMaxTwo(NUM, M);
return 0;
}
```
输出结果为:
```
The two maximum numbers are: 987, 654
```
相关推荐
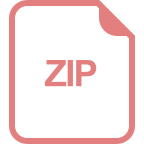
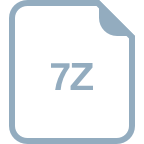
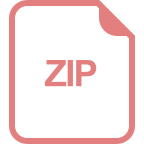














