def add_score_column(self,features_scores,col_name,col_value): # convert 1D array to rows and insert it into df colum features_scores[col_name] = pd.Series(col_value) return features_scores
时间: 2024-02-26 08:52:47 浏览: 61
这是一个定义在类中的函数,该函数的输入参数包括一个DataFrame对象features_scores,一个字符串col_name和一个列表或一维数组col_value,其作用是向features_scores中添加一个新的列,该列的列名为col_name,列的值为col_value。具体地,该函数使用pd.Series方法将一维数组col_value转换为一个行数与features_scores相同,列名为col_name的新的DataFrame对象,并将其插入到features_scores对象中,最后返回添加新列后的features_scores对象。该函数的输出是一个新的DataFrame对象features_scores,其包含了原有DataFrame对象features_scores以及新的一列。
相关问题
改进以下代码class Student: def __init__(self, num, name, scores): self.num=num self.name=name self.scores=scores def __getitem__(self, index): return self.scores[index] def __setitem__(self, index, value): self.scores[index]=value def __delitem__(self, index): del self.scores[index] def __str__(self): return self.name+"成绩为:"+self.scores zhangsan=Student("100001", "张三", [91, 87, 81.5, 69, 97, 88, 69, 78.5]) lisi=Student("100002", "李四", [95, 91, 88, 87, 97.5, 69.5, 92, 78.5]) zhangsan[0] = 102 del lisi[5:] print(zhangsan) print(lisi)
你可以尝试这样改进代码:
```
class Student:
def __init__(self, num, name, scores):
self.num = num
self.name = name
self.scores = scores
def __getitem__(self, index):
return self.scores[index]
def __setitem__(self, index, value):
self.scores[index] = value
def __delitem__(self, index):
if isinstance(index, slice):
del self.scores[index]
else:
del self.scores[index]
def __str__(self):
return f"{self.name}的成绩为:{', '.join(str(score) for score in self.scores)}"
zhangsan = Student("100001", "张三", [91, 87, 81.5, 69, 97, 88, 69, 78.5])
lisi = Student("100002", "李四", [95, 91, 88, 87, 97.5, 69.5, 92, 78.5])
zhangsan[0] = 102
del lisi[5:]
print(zhangsan)
print(lisi)
```
主要改进:
1. 在 `__delitem__` 方法中增加对 `slice` 对象的处理,以支持删除多个元素。
2. 在 `__str__` 方法中使用 `join` 拼接字符串,以更好地展示学生成绩。
3. 使用 f-string 格式化字符串,使代码更简洁易读。
def prepare_features_scores_list(self,features): features_scores = pd.DataFrame() features_scores.insert(0, 'feature', list(features)) return features_scores
这是一个定义在类中的函数,该函数的输入参数是一个DataFrame对象features,其作用是将特征列按照原有顺序生成一个新的DataFrame对象features_scores,并且在features_scores的第一列插入一个'feature'列,该列的值为原有特征列的列名。具体地,该函数首先创建一个空的DataFrame对象features_scores,然后使用insert方法在其第0列插入一个'feature'列,该列的值为原有DataFrame对象features的列名,最后返回生成的features_scores对象。该函数的输出是一个新的DataFrame对象features_scores,其包含了原有DataFrame对象features的特征列及其列名。
阅读全文
相关推荐
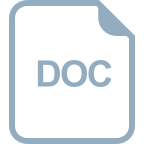
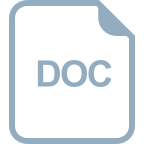
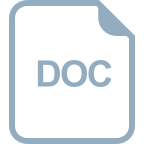














