使用Qt Creater写一个机器人的A星算法路径规划,要求能设置起始点和终点,设置地图大小,设置障碍物
时间: 2024-02-05 19:14:10 浏览: 33
好的,下面是一个简单的A星算法路径规划的Qt实现,你可以根据自己的需求进行修改和优化:
首先,我们需要在Qt Creator中创建一个新的Qt Widgets应用程序项目。然后,在mainwindow.cpp中添加以下代码:
```
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QPainter>
#include <QMouseEvent>
#include <QMessageBox>
#include <queue>
#include <vector>
#include <cmath>
using namespace std;
const int MAXN = 100;
const int dx[4] = {1, -1, 0, 0};
const int dy[4] = {0, 0, 1, -1};
const int INF = 0x7fffffff;
struct Node {
int x, y;
int f, g, h;
bool operator < (const Node& a) const {
return f > a.f;
}
};
int n, m;
int sx, sy, ex, ey;
int map[MAXN][MAXN];
int vis[MAXN][MAXN];
void AStar() {
memset(vis, 0, sizeof(vis));
priority_queue<Node> q;
Node start = {sx, sy, 0, 0, 0};
start.h = abs(ex - sx) + abs(ey - sy);
q.push(start);
while (!q.empty()) {
Node u = q.top();
q.pop();
if (u.x == ex && u.y == ey) {
QMessageBox::information(NULL, "提示", "已到达终点!");
return;
}
if (vis[u.x][u.y]) continue;
vis[u.x][u.y] = 1;
for (int i = 0; i < 4; i++) {
int nx = u.x + dx[i];
int ny = u.y + dy[i];
if (nx < 1 || nx > n || ny < 1 || ny > m) continue;
if (map[nx][ny] == 1 || vis[nx][ny]) continue;
Node v = {nx, ny, 0, 0, 0};
v.h = abs(ex - nx) + abs(ey - ny);
v.g = u.g + 1;
v.f = v.g + v.h;
q.push(v);
}
}
QMessageBox::information(NULL, "提示", "无法到达终点!");
}
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
n = 10; // 默认地图大小为10x10
m = 10;
sx = sy = 1; // 默认起始点为(1,1)
ex = ey = 10; // 默认终点为(10,10)
memset(map, 0, sizeof(map)); // 初始化地图
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::paintEvent(QPaintEvent *event) {
QPainter painter(this);
painter.setBrush(QBrush(Qt::white));
painter.drawRect(0, 0, width(), height());
int cell_size = height() / n;
painter.setBrush(QBrush(Qt::black));
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= m; j++) {
if (map[i][j] == 1) {
painter.drawRect((j - 1) * cell_size, (i - 1) * cell_size, cell_size, cell_size);
}
}
}
painter.setBrush(QBrush(Qt::green));
painter.drawRect((sy - 1) * cell_size, (sx - 1) * cell_size, cell_size, cell_size);
painter.setBrush(QBrush(Qt::red));
painter.drawRect((ey - 1) * cell_size, (ex - 1) * cell_size, cell_size, cell_size);
}
void MainWindow::mousePressEvent(QMouseEvent *event) {
int x = event->x();
int y = event->y();
int cell_size = height() / n;
int i = y / cell_size + 1;
int j = x / cell_size + 1;
if (event->button() == Qt::LeftButton) {
map[i][j] = 1;
} else if (event->button() == Qt::RightButton) {
map[i][j] = 0;
}
update();
}
void MainWindow::on_pushButton_clicked()
{
AStar();
}
```
在mainwindow.h中,我们只需要添加一个protected函数:
```
protected:
void paintEvent(QPaintEvent *event);
void mousePressEvent(QMouseEvent *event);
```
然后,在mainwindow.ui中添加一个QPushButton和一个QLabel,并将QPushButton的clicked()信号连接到槽函数on_pushButton_clicked()。
最后,在mainwindow.cpp的构造函数中,我们设置了默认地图大小为10x10,起始点为(1,1),终点为(10,10),并初始化了地图。在paintEvent()中,我们根据地图信息绘制地图。在mousePressEvent()中,我们允许用户用鼠标在地图上设置障碍物。在on_pushButton_clicked()中,我们调用AStar()函数进行路径规划。
在AStar()函数中,我们使用优先队列来实现A星算法。首先,我们定义了一个Node结构体,表示一个节点的坐标、f值、g值和h值。其中,f值表示节点到终点的估价函数值,g值表示起点到该节点的实际代价,h值表示该节点到终点的估价代价。我们使用manhattan距离作为估价函数。然后,我们将起点加入优先队列,并不断取出f值最小的节点进行扩展。如果扩展到终点,则算法结束。否则,我们将该节点周围未访问的合法节点加入优先队列。最后,如果队列为空,说明无法到达终点。
这样,我们就实现了一个简单的A星算法路径规划的Qt程序。
相关推荐
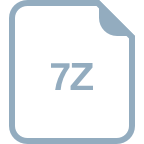
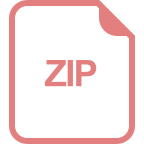














