使用sem_wait, sem_post实现共享内存读写同步, 包括进程1和进程2
时间: 2024-02-01 18:14:49 浏览: 77
下面是一个使用 sem_wait 和 sem_post 实现共享内存读写同步的示例代码,包括进程1和进程2:
进程1:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <semaphore.h>
#define SHM_KEY 1234
#define SEM_KEY 5678
typedef struct {
int value;
} shared_memory;
int main() {
int shmid = shmget(SHM_KEY, sizeof(shared_memory), IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
shared_memory *shm = shmat(shmid, NULL, 0);
if (shm == (void *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
sem_t *sem = sem_open("semaphore", O_CREAT, 0666, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
printf("Process 1 started.\n");
for (int i = 0; i < 5; i++) {
sem_wait(sem);
printf("Process 1 write %d to shared memory.\n", i);
shm->value = i;
sem_post(sem);
sleep(1);
}
sem_close(sem);
if (shmdt(shm) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
printf("Process 1 finished.\n");
return 0;
}
```
进程2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <semaphore.h>
#define SHM_KEY 1234
#define SEM_KEY 5678
typedef struct {
int value;
} shared_memory;
int main() {
int shmid = shmget(SHM_KEY, sizeof(shared_memory), IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
shared_memory *shm = shmat(shmid, NULL, 0);
if (shm == (void *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
sem_t *sem = sem_open("semaphore", O_CREAT, 0666, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
printf("Process 2 started.\n");
for (int i = 0; i < 5; i++) {
sem_wait(sem);
printf("Process 2 read %d from shared memory.\n", shm->value);
sem_post(sem);
sleep(1);
}
sem_close(sem);
if (shmdt(shm) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
printf("Process 2 finished.\n");
return 0;
}
```
在这个示例代码中,我们创建了一个共享内存区域和一个信号量用于同步读写操作。进程1和进程2都可以访问这个共享内存区域,并且在读写之前会使用信号量进行同步,保证读写操作的互斥性。
在进程1中,我们使用 sem_wait 等待信号量,然后写入数据到共享内存区域中。写入完成后,我们使用 sem_post 释放信号量。
在进程2中,我们也使用 sem_wait 等待信号量,然后从共享内存区域中读取数据。读取完成后,我们同样使用 sem_post 释放信号量。
这样,我们就实现了进程之间的共享内存读写同步。
阅读全文
相关推荐
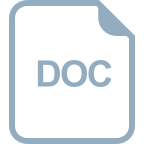
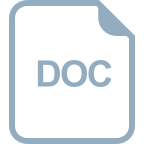
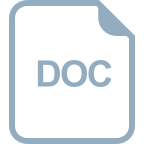











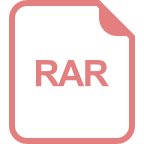
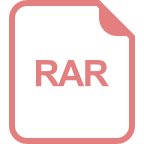
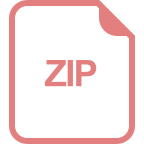
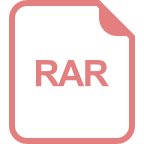

