unordered_map 使用
时间: 2023-11-30 11:42:31 浏览: 97
unordered_map是C++ STL中的一个关联容器,它提供了一种将键映射到值的方法。它的使用方法类似于map,但是它内部实现了哈希表,因此查找速度是常量级别的。以下是unordered_map的使用方法:
1. 头文件引入
```c++
#include <unordered_map>
```
2. 创建unordered_map对象
```c++
std::unordered_map<std::string, int> myMap;
```
3. 插入元素
```c++
myMap.insert(std::make_pair("apple", 1));
myMap["banana"] = 2;
```
4. 访问元素
```c++
std::cout << myMap["apple"] << std::endl; // 输出:1
```
5. 查找元素
```c++
if (myMap.find("apple") != myMap.end()) {
std::cout << "Found apple!" << std::endl;
}
```
6. 删除元素
```c++
myMap.erase("apple");
```
相关问题
unordered_map使用
unordered_map是C++ STL中的一个容器,用于实现哈希表,其可以用来存储键值对。使用unordered_map需要包含头文件<unordered_map>。
下面是unordered_map的使用示例:
```C++
#include <iostream>
#include <unordered_map>
using namespace std;
int main() {
// 创建一个unordered_map对象
unordered_map<string, int> umap;
// 插入键值对
umap.insert(make_pair("apple", 3));
umap.insert(make_pair("orange", 5));
umap["banana"] = 2;
// 访问元素
cout << "The value of apple is " << umap["apple"] << endl;
cout << "The value of orange is " << umap["orange"] << endl;
cout << "The value of banana is " << umap["banana"] << endl;
// 查找元素
if (umap.find("pear") != umap.end()) {
cout << "The value of pear is " << umap["pear"] << endl;
}
else {
cout << "Pear not found" << endl;
}
// 遍历unordered_map
for (auto it = umap.begin(); it != umap.end(); it++) {
cout << it->first << ": " << it->second << endl;
}
return 0;
}
```
输出结果:
```
The value of apple is 3
The value of orange is 5
The value of banana is 2
Pear not found
banana: 2
apple: 3
orange: 5
```
在上面的示例中,我们创建了一个字符串到整数的unordered_map对象umap,并插入了三个键值对。我们可以使用[]运算符访问元素,使用find()方法查找元素。我们还可以使用迭代器遍历unordered_map中的所有元素。
unordered_map使用示例
unordered_map是C++标准库中的一个关联容器,它提供了一种键值对的映射关系。下面是unordered_map的使用示例:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
// 创建一个unordered_map对象
std::unordered_map<std::string, int> myMap;
// 插入键值对
myMap["apple"] = 3;
myMap["banana"] = 5;
myMap["orange"] = 2;
// 访问元素
std::cout << "The number of apples is: " << myMap["apple"] << std::endl;
// 遍历unordered_map
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
// 检查某个键是否存在
if (myMap.count("banana") > 0) {
std::cout << "The key 'banana' exists in the unordered_map." << std::endl;
}
// 删除某个键值对
myMap.erase("orange");
// 清空unordered_map
myMap.clear();
return 0;
}
```
阅读全文
相关推荐
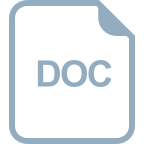
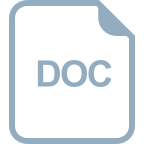
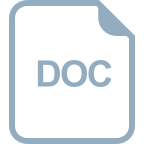




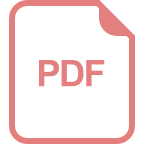







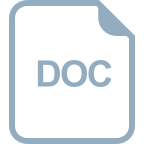
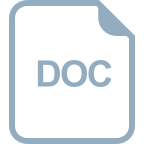