.net6 webapi获取Request.UrlReferrer,在program里面判断 UrlReferrer是否等于aaa,不等于的话断开连接,使用WebApplicationBuilder来设置,给出范例
时间: 2024-10-24 19:11:38 浏览: 14
在.NET 6 中,要创建一个 Web API 并在 `Program.cs` 中处理请求头中的 `UrlReferrer`,你需要做以下几步:
1. 首先,确保你在 `Startup.cs` 文件中设置了依赖注入以处理 HTTP 请求。添加 `Microsoft.AspNetCore.Http.Abstractions` 包。
```csharp
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
// ...
public void ConfigureServices(IServiceCollection services)
{
// 其他服务注册...
services.AddControllers();
}
```
2. 在 `Configure` 方法中,你可以选择在中间件链中拦截每个请求并检查 `UrlReferrer`。这里,我们可以自定义一个 `IEndpointFilter` 来实现这个逻辑。创建一个新的类并让它继承 `EndpointFilterAttribute`。
```csharp
using Microsoft.AspNetCore.Mvc.Filters;
public class CheckRefererFilter : IEndpointFilter
{
public void OnFiltering(Endpoint endpoint, HttpContext context)
{
if (context.Request.Headers["Referer"] != "aaa")
{
context.Response.StatusCode = StatusCodes.Status403 Forbidden;
context.Response.ContentType = "text/plain";
context.Response.Write("Access denied - referer is not 'aaa'");
context.Abort();
}
}
public async Task OnFilterAsync(Endpoint endpoint, HttpContext context, CancellationToken cancellationToken)
{
// 如果你想让同步和异步版本都执行检查,请删除此行并添加下面这行
// await OnFiltering(endpoint, context);
}
}
```
3. 将这个过滤器添加到应用的服务配置中:
```csharp
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// ...
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers().AddFilter<CheckRefererFilter>();
// 如果你想针对特定控制器或动作使用该过滤器,可以这样添加:
// endpoints.MapControllerRoute(...).AddFilter<CheckRefererFilter>();
});
}
```
现在,当请求的 `UrlReferrer` 不等于 "aaa" 时,API 将返回 403 禁止访问状态,并包含相应的错误消息。
阅读全文
相关推荐
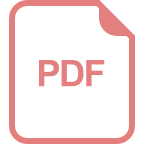
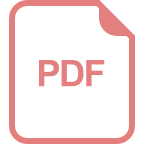
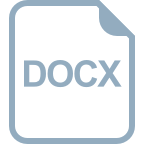




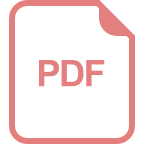
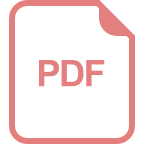
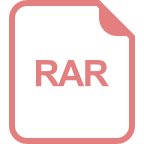
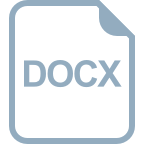
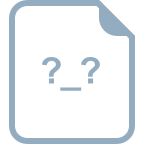
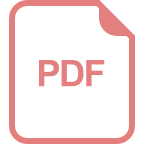
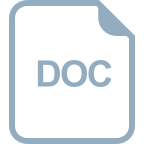
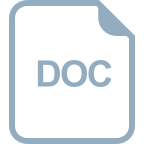
