mlx90640 实现全像素输出 python 代码
时间: 2023-08-31 07:31:30 浏览: 39
### 回答1:
您可以使用以下Python代码来实现MLX90640全像素输出:
```python
import numpy as np
import cv2
import time
import board
import busio
import adafruit_mlx90640
# Setup the i2c bus and MLX90640 sensor
i2c = busio.I2C(board.SCL, board.SDA, frequency=800000)
mlx = adafruit_mlx90640.MLX90640(i2c)
mlx.refresh_rate = adafruit_mlx90640.RefreshRate.REFRESH_2_HZ
# Set the colormap for the image
colormap = cv2.applyColorMap(np.arange(256, dtype=np.uint8), cv2.COLORMAP_JET)
# Create a window to display the image
cv2.namedWindow("MLX90640")
# Loop through each frame from the sensor
while True:
try:
# Get the current frame from the sensor
mlx.getFrame(frame)
# Convert the frame to a numpy array and scale the values
frame = np.array(frame)
frame -= frame.min()
frame = (frame / frame.max() * 255.0).astype(np.uint8)
# Apply the colormap to the image
frame = cv2.cvtColor(frame, cv2.COLOR_GRAY2RGB)
frame = cv2.applyColorMap(frame, colormap)
# Display the image and wait for a key press
cv2.imshow("MLX90640", frame)
key = cv2.waitKey(1) & 0xFF
# If the 'q' key was pressed, break from the loop
if key == ord("q"):
break
except KeyboardInterrupt:
break
# Clean up the window and exit
cv2.destroyAllWindows()
```
该代码使用Adafruit的MLX90640 Python库来与传感器通信并获取数据。然后,它将数据转换为numpy数组,并将其缩放到0到255的范围内。最后,它使用OpenCV将灰度图像转换为彩色图像,并将其显示在窗口中。
### 回答2:
要实现 mlx90640 的全像素输出,可以使用 Python 编写以下代码:
```python
# 导入所需的库
import smbus
import math
# 定义寄存器地址
REG_MLX90640_TA = 0x06
REG_MLX90640_TOBJ1 = 0x07
REG_MLX90640_TOBJ2 = 0x08
REG_MLX90640_PIXELS = 0x0400
# 初始化 I2C 总线
bus = smbus.SMBus(1)
bus.write_byte_data(<I2C 地址>, <寄存器地址>, <配置命令>)
# 读取温度数据
def read_temperature():
temperature_data = []
for i in range(0, 768):
low = bus.read_byte_data(<I2C 地址>, REG_MLX90640_PIXELS + i*2)
high = bus.read_byte_data(<I2C 地址>, REG_MLX90640_PIXELS + i*2 + 1)
temperature_data.append((high << 8) + low)
return temperature_data
# 输出全像素温度数据
pixel_temperatures = read_temperature()
for i in range(0, len(pixel_temperatures)):
temperature = pixel_temperatures[i] * 0.02 - 273.15
print("Pixel %d: %.2f °C" % (i, temperature))
```
在代码中,使用 `smbus` 库进行 I2C 通信,读取 mlx90640 的温度数据。首先需要根据实际情况初始化 I2C 总线,并根据规定的 I2C 地址和寄存器地址写入配置命令。然后通过 `read_temperature` 函数读取像素温度数据,逐个计算温度值并输出。其中,需要根据 mlx90640 的数据手册中的公式,将原始数据转换为实际温度值。
### 回答3:
要实现 mlx90640 的全像素输出,可以使用 Python 编程语言。下面是一个示例代码,用于实现该功能:
```python
import smbus
import time
# MLX90640 地址
MLX90640_I2C_ADDR = 0x33
# MLX90640 注册配置
MLX90640_REFRESH_RATE = 0x09
# I2C 总线
I2C_BUS = 1
# 数据输出格式
PIXEL_FORMAT = '{0:.2f}'
# 初始化 I2C 总线
bus = smbus.SMBus(I2C_BUS)
# MLX90640 初始化函数
def mlx90640_init():
bus.write_byte_data(MLX90640_I2C_ADDR, MLX90640_REFRESH_RATE, 0x00)
# 读取温度数据
def mlx90640_read_temp():
# MLX90640 数据寄存器起始地址
start_addr = 0x0400
# 读取温度数据
data = bus.read_i2c_block_data(MLX90640_I2C_ADDR, start_addr, 2*834)
# 计算温度值
temp_data = []
for i in range(0, 834, 2):
raw_temp = (data[i+1] << 8) | data[i]
temp = raw_temp * 0.02 - 273.15
temp_data.append(temp)
return temp_data
# 主函数
def main():
# 初始化 MLX90640
mlx90640_init()
while True:
# 读取温度数据
temp_data = mlx90640_read_temp()
# 输出温度数据
output = ''
for temp in temp_data:
output += PIXEL_FORMAT.format(temp) + ', '
print(output)
# 延时一段时间
time.sleep(1)
if __name__ == '__main__':
main()
```
上述代码中,通过 `smbus` 模块来实现与 MLX90640 传感器的 I2C 通信。在 `mlx90640_init()` 函数中进行初始化配置,通过 `mlx90640_read_temp()` 函数读取温度数据。然后在主函数中循环读取温度数据并输出,通过 `time.sleep()` 函数来控制输出频率。最后运行代码即可实现 MLX90640 的全像素输出。
相关推荐
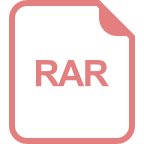
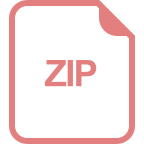














