C#读取SQLserver一个数据库里的两个表中数据整合到一个数组,并可以对数组进行排序
时间: 2023-06-17 19:06:11 浏览: 52
可以使用C#中的ADO.NET来读取SQL Server中的数据表,并将数据整合到一个数组中,然后使用Array.Sort()方法对数组进行排序。
以下是一个示例代码:
```csharp
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "<your connection string>";
string query1 = "SELECT * FROM Table1";
string query2 = "SELECT * FROM Table2";
// Create a new SqlConnection object
using (SqlConnection connection = new SqlConnection(connectionString))
{
// Open the connection
connection.Open();
// Create a new SqlCommand object for the first query
using (SqlCommand command1 = new SqlCommand(query1, connection))
{
// Execute the first query and read the data into a SqlDataReader object
using (SqlDataReader reader1 = command1.ExecuteReader())
{
// Create a new array to hold the data from both tables
int size = reader1.FieldCount;
object[,] data = new object[size, 0];
// Loop through the rows of the first table and add the data to the array
while (reader1.Read())
{
object[] row = new object[size];
for (int i = 0; i < size; i++)
{
row[i] = reader1[i];
}
Array.Resize(ref data, new int[] { size, data.GetLength(1) + 1 });
for (int i = 0; i < size; i++)
{
data[i, data.GetLength(1) - 1] = row[i];
}
}
// Create a new SqlCommand object for the second query
using (SqlCommand command2 = new SqlCommand(query2, connection))
{
// Execute the second query and read the data into a SqlDataReader object
using (SqlDataReader reader2 = command2.ExecuteReader())
{
// Loop through the rows of the second table and add the data to the array
while (reader2.Read())
{
object[] row = new object[size];
for (int i = 0; i < size; i++)
{
row[i] = reader2[i];
}
Array.Resize(ref data, new int[] { size, data.GetLength(1) + 1 });
for (int i = 0; i < size; i++)
{
data[i, data.GetLength(1) - 1] = row[i];
}
}
}
}
// Sort the array
Array.Sort(data);
// Do something with the sorted data
// ...
}
}
}
}
}
```
在上面的代码中,首先创建了一个SqlConnection对象来连接数据库,然后使用SqlCommand对象分别执行两个查询,并使用SqlDataReader对象读取数据。将数据添加到一个二维数组中,最后使用Array.Sort()方法对数组进行排序。在此之后,可以对排序后的数据做任何需要的处理。
相关推荐
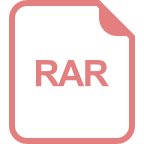
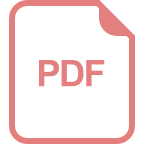














