请你帮我写一个c程序,输入十个字符,统计其中的英文字母,空格或回车,数字字符和其他字符的个数
时间: 2024-05-14 22:14:47 浏览: 35
```c
#include <stdio.h>
int main() {
char ch;
int letterCount = 0, spaceCount = 0, digitCount = 0, otherCount = 0;
printf("请输入十个字符:\n");
for (int i = 0; i < 10; i++) {
scanf(" %c", &ch); // 注意空格,避免读入回车符
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
letterCount++;
} else if (ch == ' ' || ch == '\n') {
spaceCount++;
} else if (ch >= '0' && ch <= '9') {
digitCount++;
} else {
otherCount++;
}
}
printf("字母个数:%d\n", letterCount);
printf("空格或回车个数:%d\n", spaceCount);
printf("数字个数:%d\n", digitCount);
printf("其他字符个数:%d\n", otherCount);
return 0;
}
```
相关问题
本题要求编写程序,输入10个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数。
以下是一个简单的Python程序,可以实现输入10个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数:
```python
str_input = input("请输入10个字符:")
count_letter = 0 # 统计英文字母的个数
count_space = 0 # 统计空格或回车的个数
count_digit = 0 # 统计数字字符的个数
count_other = 0 # 统计其他字符的个数
for ch in str_input:
if ch.isalpha(): # 判断是否为英文字母
count_letter += 1
elif ch.isspace(): # 判断是否为空格或回车
count_space += 1
elif ch.isdigit(): # 判断是否为数字字符
count_digit += 1
else:
count_other += 1 # 其他字符的个数
print("英文字母的个数:", count_letter)
print("空格或回车的个数:", count_space)
print("数字字符的个数:", count_digit)
print("其他字符的个数:", count_other)
```
当程序运行后,会提示用户输入10个字符,然后根据用户输入的字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数,并输出结果。
输入10个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数C语言
以下是统计字符类型个数的C语言代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c;
int letter = 0, space = 0, digit = 0, other = 0;
printf("请输入10个字符:\n");
for (int i = 0; i < 10; i++) {
c = getchar();
if (isalpha(c)) {
letter++;
} else if (isspace(c)) {
space++;
} else if (isdigit(c)) {
digit++;
} else {
other++;
}
}
printf("字母个数:%d\n空格或回车个数:%d\n数字个数:%d\n其他字符个数:%d\n", letter, space, digit, other);
return 0;
}
```
阅读全文
相关推荐
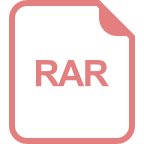













