根据MySQL与jsp生成一个购物车代码要求可以当页加减商品数量,计算商品小计与总价,可以删除清空购物车和清除商品
时间: 2024-02-16 20:03:45 浏览: 32
好的,下面是一个可以实现当页加减商品数量、计算商品小计与总价、删除、清空购物车和清除商品的购物车代码示例:
1. 商品表与用户表同上,购物车表需新增字段:`subtotal`(小计)。
2. JSP页面代码:
```
<%@ page import="java.sql.*" %>
<%
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM items");
int userId = 1;
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>商品列表</h1>
<table>
<thead>
<tr>
<th>名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<% while(rs.next()) { %>
<tr>
<td><%= rs.getString("name") %></td>
<td><%= rs.getDouble("price") %></td>
<td>
<form method="post" action="updateCart.jsp">
<input type="hidden" name="itemId" value="<%= rs.getInt("id") %>">
<input type="hidden" name="action" value="update">
<input type="number" name="quantity" value="1" min="1" max="99">
<input type="submit" value="更新">
</form>
</td>
<td><%= rs.getDouble("price") %></td>
<td>
<form method="post" action="updateCart.jsp">
<input type="hidden" name="itemId" value="<%= rs.getInt("id") %>">
<input type="hidden" name="action" value="delete">
<input type="submit" value="删除">
</form>
</td>
</tr>
<% } %>
</tbody>
</table>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<%
double total = 0;
ResultSet cartRs = stmt.executeQuery("SELECT * FROM cart WHERE user_id = " + userId);
while(cartRs.next()) {
int itemId = cartRs.getInt("item_id");
int quantity = cartRs.getInt("quantity");
double subtotal = cartRs.getDouble("subtotal");
ResultSet itemRs = stmt.executeQuery("SELECT * FROM items WHERE id = " + itemId);
itemRs.next();
String itemName = itemRs.getString("name");
double itemPrice = itemRs.getDouble("price");
total += subtotal;
%>
<tr>
<td><%= itemName %></td>
<td><%= itemPrice %></td>
<td>
<form method="post" action="updateCart.jsp">
<input type="hidden" name="itemId" value="<%= itemId %>">
<input type="hidden" name="action" value="update">
<input type="number" name="quantity" value="<%= quantity %>" min="1" max="99">
<input type="submit" value="更新">
</form>
</td>
<td><%= subtotal %></td>
<td>
<form method="post" action="updateCart.jsp">
<input type="hidden" name="itemId" value="<%= itemId %>">
<input type="hidden" name="action" value="delete">
<input type="submit" value="删除">
</form>
</td>
</tr>
<% } %>
<tr>
<td colspan="3">总计:</td>
<td><%= total %></td>
<td>
<form method="post" action="updateCart.jsp">
<input type="hidden" name="action" value="clear">
<input type="submit" value="清空购物车">
</form>
</td>
</tr>
</tbody>
</table>
</body>
</html>
```
3. `updateCart.jsp`代码:
```
<%@ page import="java.sql.*" %>
<%
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
Statement stmt = conn.createStatement();
int userId = 1;
int itemId = Integer.parseInt(request.getParameter("itemId"));
String action = request.getParameter("action");
if ("update".equals(action)) {
int quantity = Integer.parseInt(request.getParameter("quantity"));
double subtotal = 0;
ResultSet itemRs = stmt.executeQuery("SELECT price FROM items WHERE id = " + itemId);
itemRs.next();
double itemPrice = itemRs.getDouble("price");
subtotal = itemPrice * quantity;
PreparedStatement pstmt = conn.prepareStatement("UPDATE cart SET quantity = ?, subtotal = ? WHERE user_id = ? AND item_id = ?");
pstmt.setInt(1, quantity);
pstmt.setDouble(2, subtotal);
pstmt.setInt(3, userId);
pstmt.setInt(4, itemId);
pstmt.executeUpdate();
} else if ("delete".equals(action)) {
PreparedStatement pstmt = conn.prepareStatement("DELETE FROM cart WHERE user_id = ? AND item_id = ?");
pstmt.setInt(1, userId);
pstmt.setInt(2, itemId);
pstmt.executeUpdate();
} else if ("clear".equals(action)) {
PreparedStatement pstmt = conn.prepareStatement("DELETE FROM cart WHERE user_id = ?");
pstmt.setInt(1, userId);
pstmt.executeUpdate();
}
response.sendRedirect("cart.jsp");
%>
```
上面的代码实现了当页加减商品数量、计算商品小计与总价、删除、清空购物车和清除商品功能。需要注意的是,这只是一个示例,实际情况中需要根据你的具体需求进行调整和完善。
相关推荐
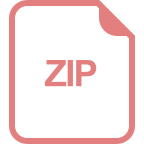














