img_color[:, :, i] = np.divide(img[:, :, i], img_sum_expanded) ValueError: operands could not be broadcast together with shapes (480,640) (480,640,1,3)
时间: 2023-07-16 19:15:41 浏览: 113
这个错误是因为 `img_sum_expanded` 是一个四维数组,而 `img[:, :, i]` 是一个二维数组,它们的形状不一样,无法直接进行相除操作。
你可以尝试将 `img[:, :, i]` 扩展一下维度,使其变成一个四维数组,形状与 `img_sum_expanded` 一致,然后再进行相除操作。以下是一种可能的实现方式:
```python
img_color = np.zeros_like(img)
img_sum_expanded = np.expand_dims(img_sum, axis=-1)
img_sum_expanded = np.expand_dims(img_sum_expanded, axis=-1)
img_sum_expanded = np.expand_dims(img_sum_expanded, axis=-1)
for i in range(3):
img_color[:, :, i] = np.divide(img[:, :, i][:, :, np.newaxis, np.newaxis], img_sum_expanded)
```
这里先使用 `np.expand_dims` 函数将 `img_sum` 扩展三次,使其从二维变成了四维,然后用 `img[:, :, i][:, :, np.newaxis, np.newaxis]` 将 `img[:, :, i]` 扩展两次,使其也变成了四维。这样就可以进行相除操作了。
相关问题
完善以下代码norm = np.sum(mf_out, axis=2, keepdims=True) '''print(mf_out) print(norm)''' # mf_norm = np.divide(mf_out, norm) my_norm = [] for i in norm: if i == 0: break else: mf_norm = np.divide(mf_out, norm) my_norm.append(mf_norm) #mf_norm = mf_out / norm '''print(mf_norm)''' # Flatten normalized MFs for input to output layer flat = mf_norm.reshape(len(X_batch), -1)
好的,如果您想要在计算 `mf_norm` 时,将除数为 0 的元素替换为 1,可以使用如下代码:
```python
import numpy as np
norm = np.sum(mf_out, axis=2, keepdims=True)
mf_norm = np.divide(mf_out, np.where(norm == 0, 1, norm))
my_norm = []
for i in range(len(norm)):
if norm[i] == 0:
my_norm.append(np.zeros_like(mf_out[i]))
else:
my_norm.append(mf_norm[i])
flat = np.array(my_norm).reshape(len(X_batch), -1)
```
上述代码中,我们首先计算了 `norm`,然后使用 `np.where()` 函数判断 `norm` 中是否有元素为 0,如果是,则将其替换为 1,否则不作处理。接着,使用 `np.divide()` 函数计算 `mf_norm`,并保存到一个新的列表 `my_norm` 中。在循环中,我们使用 `range(len(norm))` 来遍历 `norm` 中的每个元素,如果该元素为 0,则将一个与 `mf_out` 相同形状的全零数组添加到 `my_norm` 中,否则将 `mf_norm` 中对应位置的元素添加到 `my_norm` 中。
最后,使用 `np.array()` 将 `my_norm` 转换为 NumPy 数组,并使用 `reshape()` 函数将其展平为二维数组 `flat`,以便进行下一步的操作。
Traceback (most recent call last): File "/media/linux/643A-4CF3/code/SelfDeblur-master/hsizengqiang2.py", line 70, in <module> enhanced_image = MSRCR(image) File "/media/linux/643A-4CF3/code/SelfDeblur-master/hsizengqiang2.py", line 41, in MSRCR img_color[:, :, i] = np.divide(img[:, :, i], img_sum) ValueError: operands could not be broadcast together with shapes (480,640) (480,640,3)
这个错误是因为在MSRCR函数中,img_sum是一个(480,640)的数组,而img_color是一个(480,640,3)的数组。在尝试将img[:, :, i]除以img_sum时,两者的形状不匹配,导致了这个错误。你可以先使用np.expand_dims()函数将img_sum的维度扩展为(480,640,3),然后再进行除法操作。例如:
```
img_sum_expanded = np.expand_dims(img_sum, axis=2)
img_color = np.zeros_like(img)
for i in range(3):
img_color[:, :, i] = np.divide(img[:, :, i], img_sum_expanded)
```
这样就可以避免这个错误了。
阅读全文
相关推荐
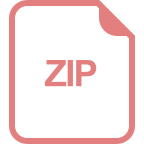
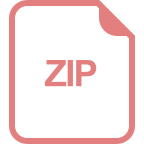
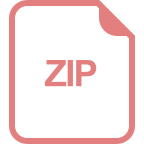








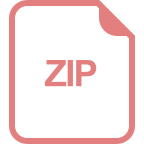


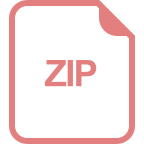
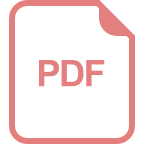
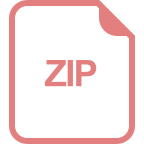