用pytorch写一个使用GPU对MLP模型批量调参的代码
时间: 2023-06-02 16:08:27 浏览: 163
可以的,以下是使用GPU对MLP模型批量调参的代码示例:
```
import torch
from sklearn.model_selection import GridSearchCV
from sklearn.neural_network import MLPRegressor
# 加载数据集
X_train, y_train = load_data()
# 定义参数搜索空间
param_grid = {
'hidden_layer_sizes': [(10,), (50,), (100,), (10, 10), (50, 50), (100, 100)],
'activation': ['relu', 'tanh', 'logistic'],
'solver': ['sgd', 'adam']
}
# 创建模型实例
mlp = MLPRegressor()
# 使用grid search进行参数调优
grid_search = GridSearchCV(mlp, param_grid=param_grid, cv=3, n_jobs=-1)
grid_search.fit(X_train, y_train)
# 打印最优参数
print("Best parameters: ", grid_search.best_params_)
# 使用最优参数创建最终模型
mlp = MLPRegressor(**grid_search.best_params_)
# 将数据装入GPU
X_train = torch.tensor(X_train).float().cuda()
y_train = torch.tensor(y_train).float().cuda()
# 将模型装入GPU
mlp = mlp.cuda()
# 训练模型
mlp.fit(X_train, y_train)
# 将模型从GPU装回CPU
mlp = mlp.cpu()
# 预测
y_pred = mlp.predict(X_test)
```
需要注意的是,这里用到了sklearn库的GridSearchCV函数来进行参数搜索,但是该函数默认使用CPU,如果要使用GPU加速,需要把n_jobs参数设为-1,具体说明详见sklearn文档。除此之外,还需要用torch.tensor()将数据从numpy array转换为pytorch tensor,并且把模型装入GPU使用mlp.cuda(),训练完成后再将模型装入CPU使用mlp.cpu()。
阅读全文
相关推荐
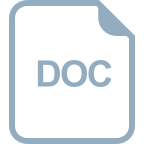
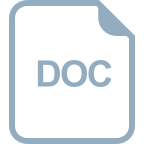
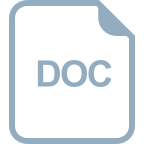
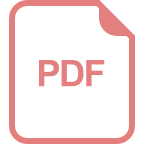
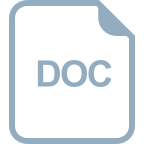
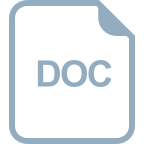
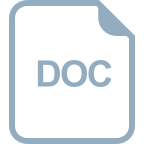
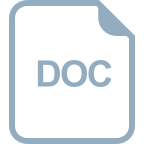
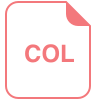
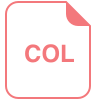
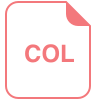
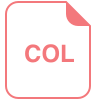
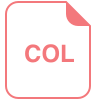
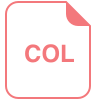
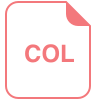
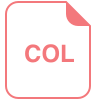
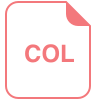
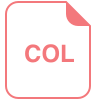
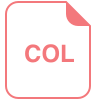