JAVA编写扫雷游戏的代码
时间: 2023-10-30 11:06:45 浏览: 85
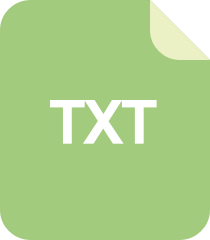
java扫雷游戏代码
当然可以。以下是Java编写的扫雷游戏的代码示例:
```java
import java.util.Scanner;
import java.util.Random;
public class Minesweeper {
public static void main(String[] args) {
int rows = 10; // 游戏界面的行数
int cols = 10; // 游戏界面的列数
int bombs = 10; // 雷的数量
Scanner scanner = new Scanner(System.in);
Random random = new Random();
int[][] board = new int[rows][cols]; // 存放游戏界面的数字
boolean[][] revealed = new boolean[rows][cols]; // 记录每个方格是否已经被揭示
// 将所有方格的数字初始化为0
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j] = 0;
}
}
// 在随机位置放置雷
int count = 0;
while (count < bombs) {
int i = random.nextInt(rows);
int j = random.nextInt(cols);
if (board[i][j] == 0) {
board[i][j] = -1; // -1表示这个方格是雷
count++;
}
}
// 计算每个非雷方格周围的雷的数量
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (board[i][j] == 0) {
int numBombs = 0;
for (int di = -1; di <= 1; di++) {
for (int dj = -1; dj <= 1; dj++) {
int ni = i + di;
int nj = j + dj;
if (ni >= 0 && ni < rows && nj >= 0 && nj < cols && board[ni][nj] == -1) {
numBombs++;
}
}
}
board[i][j] = numBombs;
}
}
}
// 开始游戏循环
while (true) {
// 打印游戏界面
System.out.println(" 0123456789");
System.out.println(" ----------");
for (int i = 0; i < rows; i++) {
System.out.print(i + "|");
for (int j = 0; j < cols; j++) {
if (revealed[i][j]) {
if (board[i][j] == -1) {
System.out.print("*");
} else {
System.out.print(board[i][j]);
}
} else {
System.out.print(".");
}
}
System.out.println("|" + i);
}
System.out.println(" ----------");
// 让用户输入下一步操作
System.out.print("Enter row and column: ");
int row = scanner.nextInt();
int col = scanner.nextInt();
String action = scanner.nextLine().trim();
if (action.equals("flag")) {
// 标记一个方格是雷
if (!revealed[row][col]) {
if (board[row][col] == -1) {
System.out.println("Correct!");
revealed[row][col] = true;
} else {
System.out.println("Incorrect!");
}
} else {
System.out.println("Already revealed!");
}
} else if (action.equals("reveal")) {
// 揭示一个方格
if (!revealed[row][col]) {
if (board[row][col] == -1) {
System.out.println("Game over!");
return;
} else {
System.out.println("Correct!");
revealed[row][col] = true;
}
} else {
System.out.println("Already revealed!");
}
} else {
System.out.println("Unknown action!");
}
// 检查游戏是否结束
boolean allRevealed = true;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (!revealed[i][j]) {
allRevealed = false;
break;
}
}
}
if (allRevealed) {
System.out.println("You win!");
return;
}
}
}
}
```
这是一个基于控制台的简单扫雷游戏实现,你可以根据需要进行修改和优化。
阅读全文
相关推荐
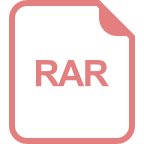
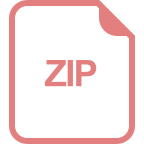
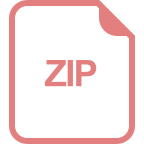
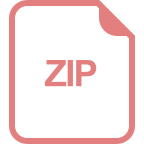
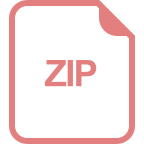
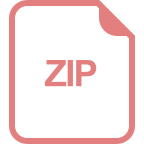