用java + fxml 做一个串口调试助手,并能够在输入框输入十六进制S/N码并通过串口存入,在fxml显示
时间: 2024-03-03 10:49:31 浏览: 71
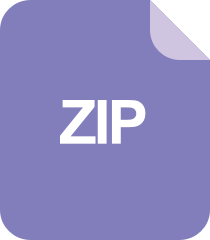
使用LABview2018制作的串口调试助手,支持十六进制、文件发送等功能

下面是一个使用JavaFX和Java Comm API实现的串口调试助手,可以在输入框中输入十六进制S/N码并通过串口存入,并在界面上显示串口发送和接收的数据:
```java
import gnu.io.CommPortIdentifier;
import gnu.io.SerialPort;
import javafx.application.Platform;
import javafx.fxml.FXML;
import javafx.scene.control.*;
import javafx.scene.input.KeyCode;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Enumeration;
public class Controller {
@FXML
private TextArea logTextArea;
@FXML
private TextField sendTextField;
@FXML
private CheckBox hexCheckBox;
@FXML
private Label statusLabel;
private SerialPort serialPort;
private InputStream inputStream;
private OutputStream outputStream;
public void initialize() {
// 监听串口数据
Thread thread = new Thread(() -> {
byte[] buffer = new byte[1024];
int len;
try {
while (true) {
if (inputStream != null && (len = inputStream.read(buffer)) > 0) {
String data = new String(buffer, 0, len);
Platform.runLater(() -> logTextArea.appendText(data));
}
}
} catch (IOException e) {
e.printStackTrace();
}
});
thread.setDaemon(true);
thread.start();
}
public void connectSerialPort() {
Enumeration portList = CommPortIdentifier.getPortIdentifiers();
while (portList.hasMoreElements()) {
CommPortIdentifier portIdentifier = (CommPortIdentifier) portList.nextElement();
if (portIdentifier.getPortType() == CommPortIdentifier.PORT_SERIAL) {
String name = portIdentifier.getName();
try {
SerialPort serialPort = (SerialPort) portIdentifier.open("SerialPort", 2000);
serialPort.setSerialPortParams(9600, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE);
inputStream = serialPort.getInputStream();
outputStream = serialPort.getOutputStream();
this.serialPort = serialPort;
statusLabel.setText(name + "已连接");
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
public void disconnectSerialPort() {
if (serialPort != null) {
serialPort.close();
serialPort = null;
statusLabel.setText("串口已断开");
}
}
public void send() {
String data = sendTextField.getText();
if (hexCheckBox.isSelected()) {
data = data.replaceAll("\\s+", "");
byte[] bytes = hexStringToBytes(data);
if (bytes != null && bytes.length > 0) {
try {
outputStream.write(bytes);
outputStream.flush();
logTextArea.appendText("发送: " + data + "\n");
sendTextField.clear();
} catch (IOException e) {
e.printStackTrace();
}
}
} else {
try {
outputStream.write(data.getBytes());
outputStream.flush();
logTextArea.appendText("发送: " + data + "\n");
sendTextField.clear();
} catch (IOException e) {
e.printStackTrace();
}
}
}
private byte[] hexStringToBytes(String hexString) {
if (hexString == null || hexString.equals("")) {
return null;
}
hexString = hexString.toUpperCase();
int length = hexString.length() / 2;
char[] hexChars = hexString.toCharArray();
byte[] bytes = new byte[length];
for (int i = 0; i < length; i++) {
int pos = i * 2;
bytes[i] = (byte) (charToByte(hexChars[pos]) << 4 | charToByte(hexChars[pos + 1]));
}
return bytes;
}
private byte charToByte(char c) {
return (byte) "0123456789ABCDEF".indexOf(c);
}
public void clearLog() {
logTextArea.clear();
}
public void onSendTextFieldKeyPressed(javafx.scene.input.KeyEvent keyEvent) {
if (keyEvent.getCode() == KeyCode.ENTER) {
send();
}
}
}
```
在FXML文件中,使用TextArea、TextField、CheckBox和Label分别绑定对应的方法即可实现功能。需要注意的是,在连接串口时需要将串口通信参数修改为实际的参数。另外,在发送数据时可以选择将数据转换为十六进制格式。
阅读全文
相关推荐
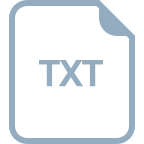
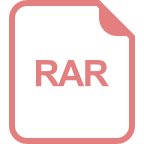


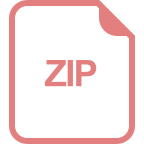
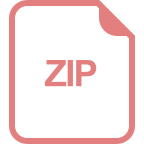
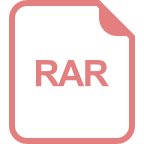
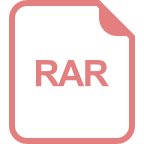








