帮我写一个网页键盘,我有四个输入框,能够输入二进制、八进制、十进制和十六进制,键盘可以选择向这四个输入框输入,当输入其中一个,可以改变其他三个的内容
时间: 2024-03-24 08:41:52 浏览: 147
好的,我可以帮你实现一个基于JavaScript的网页键盘,满足你的需求。
首先,我们需要在 HTML 中添加四个输入框和一个键盘按钮。代码如下:
```html
<input type="text" id="binary" placeholder="二进制">
<input type="text" id="octal" placeholder="八进制">
<input type="text" id="decimal" placeholder="十进制">
<input type="text" id="hexadecimal" placeholder="十六进制">
<button onclick="showKeyboard()">键盘</button>
```
接下来,我们需要编写 JavaScript 代码,实现键盘的功能。我们可以创建一个包含数字和字母的键盘,当点击键盘上的按钮时,将对应的字符插入到当前选中的输入框中。
```javascript
// 定义键盘字符
var keyboardChars = [
'0', '1', '2', '3', '4', '5', '6', '7', '8', '9',
'A', 'B', 'C', 'D', 'E', 'F'
];
// 获取输入框元素
var binaryInput = document.getElementById('binary');
var octalInput = document.getElementById('octal');
var decimalInput = document.getElementById('decimal');
var hexadecimalInput = document.getElementById('hexadecimal');
// 定义键盘显示函数
function showKeyboard() {
// 创建键盘元素
var keyboard = document.createElement('div');
keyboard.id = 'keyboard';
// 添加键盘按钮
for (var i = 0; i < keyboardChars.length; i++) {
var button = document.createElement('button');
button.innerText = keyboardChars[i];
button.onclick = function() {
var input = document.activeElement;
input.value += this.innerText;
updateInputs();
};
keyboard.appendChild(button);
}
// 显示键盘
document.body.appendChild(keyboard);
}
// 定义更新输入框函数
function updateInputs() {
var binary = binaryInput.value;
var octal = parseInt(binary, 2).toString(8);
var decimal = parseInt(binary, 2).toString(10);
var hexadecimal = parseInt(binary, 2).toString(16).toUpperCase();
octalInput.value = octal;
decimalInput.value = decimal;
hexadecimalInput.value = hexadecimal;
}
```
在代码中,我们定义了一个包含数字和字母的键盘,当点击键盘上的按钮时,将对应的字符插入到当前选中的输入框中。同时,我们还定义了一个更新输入框的函数,根据二进制输入框中的内容更新其他三个输入框的内容。
最后,我们还需要为键盘元素添加样式,让它看起来更像一个真正的键盘。
```css
#keyboard {
position: fixed;
bottom: 0;
left: 0;
width: 100%;
height: 200px;
background: #333;
text-align: center;
font-size: 30px;
}
#keyboard button {
display: inline-block;
width: 50px;
height: 50px;
margin: 10px;
border: none;
border-radius: 5px;
background: #666;
color: #fff;
font-size: 24px;
cursor: pointer;
}
```
完整的代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>网页键盘</title>
<style type="text/css">
#keyboard {
position: fixed;
bottom: 0;
left: 0;
width: 100%;
height: 200px;
background: #333;
text-align: center;
font-size: 30px;
}
#keyboard button {
display: inline-block;
width: 50px;
height: 50px;
margin: 10px;
border: none;
border-radius: 5px;
background: #666;
color: #fff;
font-size: 24px;
cursor: pointer;
}
</style>
</head>
<body>
<input type="text" id="binary" placeholder="二进制">
<input type="text" id="octal" placeholder="八进制">
<input type="text" id="decimal" placeholder="十进制">
<input type="text" id="hexadecimal" placeholder="十六进制">
<button onclick="showKeyboard()">键盘</button>
<script type="text/javascript">
// 定义键盘字符
var keyboardChars = [
'0', '1', '2', '3', '4', '5', '6', '7', '8', '9',
'A', 'B', 'C', 'D', 'E', 'F'
];
// 获取输入框元素
var binaryInput = document.getElementById('binary');
var octalInput = document.getElementById('octal');
var decimalInput = document.getElementById('decimal');
var hexadecimalInput = document.getElementById('hexadecimal');
// 定义键盘显示函数
function showKeyboard() {
// 创建键盘元素
var keyboard = document.createElement('div');
keyboard.id = 'keyboard';
// 添加键盘按钮
for (var i = 0; i < keyboardChars.length; i++) {
var button = document.createElement('button');
button.innerText = keyboardChars[i];
button.onclick = function() {
var input = document.activeElement;
input.value += this.innerText;
updateInputs();
};
keyboard.appendChild(button);
}
// 显示键盘
document.body.appendChild(keyboard);
}
// 定义更新输入框函数
function updateInputs() {
var binary = binaryInput.value;
var octal = parseInt(binary, 2).toString(8);
var decimal = parseInt(binary, 2).toString(10);
var hexadecimal = parseInt(binary, 2).toString(16).toUpperCase();
octalInput.value = octal;
decimalInput.value = decimal;
hexadecimalInput.value = hexadecimal;
}
</script>
</body>
</html>
```
阅读全文
相关推荐
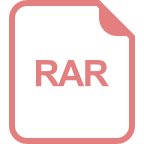
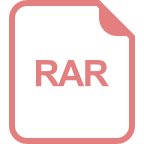
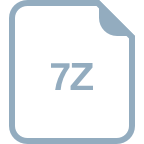















