使用所学知识编写一个基于控制台的java购书系统,实现购书功能。输出所有图书的信息:包括每本书的编号、书名、单价、库存。 顾客购买书时,根据提示输入图书编号来选购需要的书,并根据提示输入购买书的的数量。 购买完毕后输出顾客的订单信息,包括:订单号、订单明细、订单总额。
时间: 2024-12-19 07:14:18 浏览: 10
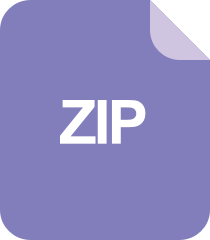
开发基于控制台的购书系统_java源代码.doc
这是一个简单的命令行交互式的Java购书系统设计概述:
```java
import java.util.HashMap;
import java.util.Map;
class Book {
private int id;
private String title;
private double price;
private int stock;
public Book(int id, String title, double price, int stock) {
this.id = id;
this.title = title;
this.price = price;
this.stock = stock;
}
// getter和setter方法省略
@Override
public String toString() {
return "Book{" +
"id=" + id +
", title='" + title + '\'' +
", price=" + price +
", stock=" + stock +
'}';
}
}
class ShoppingCart {
private Map<Integer, Integer> cart = new HashMap<>();
public void addBook(Book book, int quantity) {
if (book.getStock() >= quantity) {
cart.put(book.getId(), quantity);
book.setStock(book.getStock() - quantity);
} else {
System.out.println("Sorry, not enough stock for book " + book.getTitle());
}
}
// 提供获取购物车详情的方法
public void printCart() {
System.out.println("Order Details:");
for (Map.Entry<Integer, Integer> entry : cart.entrySet()) {
System.out.printf("Book %d: %s x %d\n", entry.getKey(), entry.getValue(), bookById(entry.getKey()).toString());
}
calculateTotal();
}
private Book bookById(int id) {
// 实现从ID查找Book对象的功能,这里假设有一个BookList存储所有书籍
// 如果库中有这本书,则返回;反之返回null
}
private void calculateTotal() {
double total = 0;
for (int quantity : cart.values()) {
total += quantity * bookById(cart.keySet().iterator().next()).price;
}
System.out.println("Order Total: " + total);
}
}
public class Main {
public static void main(String[] args) {
// 初始化一些书籍数据
Book book1 = new Book(1, "Book1", 50.0, 100);
Book book2 = new Book(2, "Book2", 70.0, 80);
// 创建购物车
ShoppingCart cart = new ShoppingCart();
// 用户选择购书操作,输入图书编号和数量
while (true) {
System.out.println("Enter book ID and quantity to buy, or type 'quit' to exit:");
String input = scanner.nextLine();
if ("quit".equals(input)) break;
String[] parts = input.split(" ");
int id = Integer.parseInt(parts[0]);
int quantity = Integer.parseInt(parts[1]);
// 检查并添加到购物车
cart.addBook(bookById(id), quantity);
}
// 显示订单信息
cart.printCart();
}
}
```
在这个例子中,我们首先定义了`Book`类表示图书信息,`ShoppingCart`类用于管理用户的购物车和结算过程。在`main`函数中,用户通过循环输入图书编号和数量来选购,最后显示订单信息。
阅读全文
相关推荐
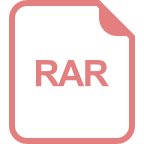
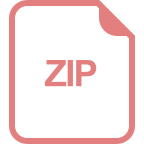










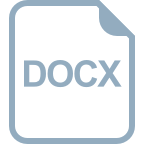

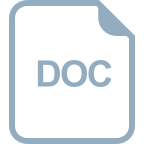
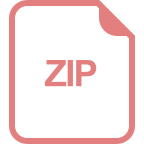
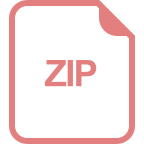
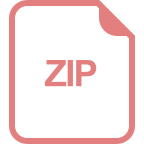