定义一个点Point类,有点坐标属性(x, y),再定义一个矩形Rectangle类,其属性为两个Point点(分别表示矩形对角线上两点的坐标),及含有计算矩形的周长及面积的成员函数;再编写两个Point类的友元函数,分别计算两个点组成的矩形的周长与面积。在主函数中进行测试。
时间: 2024-03-26 16:41:16 浏览: 41
好的,这是一个很好的面向对象编程的问题,我会给你一个基本的代码框架,你可以在此基础上进行修改和完善。
```
#include <iostream>
#include <cmath>
using namespace std;
class Point {
public:
Point(double x = 0, double y = 0) : x(x), y(y) {}
double getX() const { return x; }
double getY() const { return y; }
private:
double x, y;
friend class Rectangle;
friend double distance(Point &a, Point &b);
friend double area(Point &a, Point &b);
};
class Rectangle {
public:
Rectangle(Point p1, Point p2) : p1(p1), p2(p2) {}
double getCircumference() const {
double length = abs(p1.getX() - p2.getX());
double width = abs(p1.getY() - p2.getY());
return 2 * (length + width);
}
double getArea() const {
double length = abs(p1.getX() - p2.getX());
double width = abs(p1.getY() - p2.getY());
return length * width;
}
private:
Point p1, p2;
};
double distance(Point &a, Point &b) {
double dx = a.x - b.x;
double dy = a.y - b.y;
return sqrt(dx * dx + dy * dy);
}
double area(Point &a, Point &b) {
double length = abs(a.getX() - b.getX());
double width = abs(a.getY() - b.getY());
return length * width;
}
int main() {
Point p1(1, 2), p2(3, 4);
Rectangle rect(p1, p2);
cout << "Circumference: " << rect.getCircumference() << endl;
cout << "Area: " << rect.getArea() << endl;
cout << "Distance: " << distance(p1, p2) << endl;
cout << "Area: " << area(p1, p2) << endl;
return 0;
}
```
在主函数中,我们定义了两个点(p1和p2),然后用它们创建了一个矩形实例(rect)。接着,我们调用了Rectangle类中的getCircumference()和getArea()函数,以获取矩形的周长和面积。
我们还编写了两个友元函数,分别计算由两个点组成的矩形的周长和面积。友元函数可以访问类的私有成员,因此可以轻松地计算矩形的周长和面积。
最后,我们在主函数中打印了矩形的周长和面积,以及两个点之间的距离和由这两个点组成的矩形的面积。
阅读全文
相关推荐
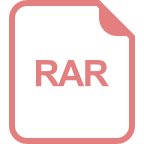









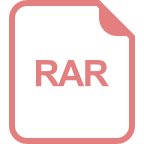
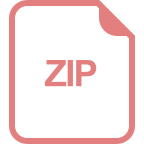