//复制目录 public static void copyDirectory(File in, File out){ //判断目标目录是否存在 if (!out.exists()) { //目标目录不存在,创建此目录 out.mkdir(); } //获取目标目录绝对路径 String path = out.getPath(); //获取源目录下的所有目录、文件,并遍历 File[] inFiles = in.listFiles(); for (File file : inFiles) { //更改目标路径 out = new File(path, file.getName()); //判断file是否为目录, //如果是目录则复制此目录,如果不是则复制此文件 if (file.isDirectory()) { copyDirectory(file, out); } else { copyFile(file, out); } } }
时间: 2024-03-11 19:44:49 浏览: 51
这是一个 Java 代码片段,函数名为copyDirectory,实现的功能是复制一个目录及其子目录和文件到另一个目录中。该函数接受两个参数,一个是源目录in,一个是目标目录out。函数先判断目标目录是否存在,如果不存在则创建该目录。然后遍历源目录下的所有目录和文件,如果是目录则递归调用copyDirectory函数,如果是文件则调用copyFile函数进行复制。
相关问题
public static void copy(File file, File toFile) throws Exception { String s = toFile.getAbsolutePath().replaceAll("\\\\", "/"); int i = s.lastIndexOf("/"); String filePath = s.substring(0,i); File fileDirectory = new File(filePath); //新建文件夹 if(!fileDirectory.exists()){ fileDirectory.mkdirs(); } byte[] b = new byte[1024]; int a; FileInputStream fis; FileOutputStream fos; //写文件 fis = new FileInputStream(file); fos = new FileOutputStream(toFile); while ((a = fis.read(b)) != -1) { fos.write(b, 0, a); } } 此方法提示拒绝访问,是为什么
这个方法提示拒绝访问的原因可能有几种可能性:
1. 权限不足:在执行文件复制操作时,可能当前用户没有足够的权限来读取或写入文件。你可以检查一下文件的权限设置,确保当前用户具有读取和写入文件的权限。
2. 文件被占用:如果文件正在被其他程序或进程使用,你可能无法复制它。在执行复制操作之前,确定文件没有被其他程序锁定或打开。
3. 文件不存在:当源文件不存在时,复制操作会失败并提示拒绝访问。你可以确保源文件存在并且路径正确。
4. 文件或目录名包含非法字符:在某些操作系统中,文件或目录名包含一些特殊字符可能会导致访问被拒绝。确保文件和目录名符合操作系统的命名规范。
这些是一些常见的原因,导致方法提示拒绝访问。你可以仔细检查代码和文件的相关设置,以确定具体的原因。
用java实现以下程序: 复制文件D://pic//chun.jpg到C://img目录中(需要先建立相关的目录和文件) 。
### 回答1:
以下是用Java实现复制文件的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileCopyExample {
public static void main(String[] args) {
String sourceFilePath = "D://pic//chun.jpg";
String destinationDirectoryPath = "C://img";
File sourceFile = new File(sourceFilePath);
File destinationDirectory = new File(destinationDirectoryPath);
if (!destinationDirectory.exists()) {
destinationDirectory.mkdirs();
}
try (FileInputStream inputStream = new FileInputStream(sourceFile);
FileOutputStream outputStream = new FileOutputStream(destinationDirectoryPath + "//" + sourceFile.getName())) {
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
System.out.println("File copied successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
示例代码中,首先定义了源文件路径和目标文件夹路径,接着使用File类创建源文件和目标文件夹的实例。如果目标文件夹不存在,则使用mkdirs()方法创建目标文件夹。
在try-with-resources语句块中,创建FileInputStream和FileOutputStream的实例,分别读取源文件和写入目标文件。使用byte数组缓冲读取数据,并使用write方法写入数据。最后,在finally块中关闭输入输出流。
执行程序后,源文件将被复制到指定的目标文件夹中。
### 回答2:
使用Java实现复制文件的操作可以使用Java的IO流来实现,具体步骤如下:
1. 先创建相关的目录和文件
可以使用Java的File类来创建目录和文件,具体代码如下:
```java
File directory = new File("C://img");
if (!directory.exists()) {
directory.mkdirs(); // 创建目录
}
File file = new File("C://pic//chun.jpg");
if (!file.exists()) {
file.createNewFile(); // 创建文件
}
```
2. 复制文件
可以使用Java的FileInputStream和FileOutputStream来实现文件的复制,具体代码如下:
```java
File srcFile = new File("D://pic//chun.jpg");
File dstFile = new File("C://img//chun_copy.jpg");
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(dstFile);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fis.close();
fos.close();
```
以上代码实现了将D://pic//chun.jpg文件复制到C://img目录中,并创建了相关的目录和文件。
### 回答3:
首先,需要使用java.io包中的File类来创建相关的目录和文件。可以按照以下步骤实现该程序:
1. 使用File类的mkdirs方法来创建目录:C://img。
```java
File dir = new File("C://img");
dir.mkdirs();
```
2. 使用File类的createNewFile方法来创建文件:C://img//chun.jpg。
```java
File file = new File("C://img//chun.jpg");
file.createNewFile();
```
3. 使用java.nio包中的Files类的copy方法来复制文件:
```java
Path source = Paths.get("D://pic//chun.jpg");
Path destination = Paths.get("C://img//chun.jpg");
Files.copy(source, destination, StandardCopyOption.REPLACE_EXISTING);
```
其中,source表示源文件路径,destination表示目标文件路径,StandardCopyOption.REPLACE_EXISTING表示如果目标文件已存在,将被替换。
4. 完整的程序如下:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
public class FileCopyExample {
public static void main(String[] args) {
try {
// 创建目录
File dir = new File("C://img");
dir.mkdirs();
// 创建文件
File file = new File("C://img//chun.jpg");
file.createNewFile();
// 复制文件
Path source = Paths.get("D://pic//chun.jpg");
Path destination = Paths.get("C://img//chun.jpg");
Files.copy(source, destination, StandardCopyOption.REPLACE_EXISTING);
System.out.println("文件复制成功!");
} catch (IOException e) {
System.out.println("发生错误:" + e.getMessage());
}
}
}
```
此程序会将D://pic目录下的chun.jpg文件复制到C://img目录中,并在控制台输出"文件复制成功!"。
阅读全文
相关推荐
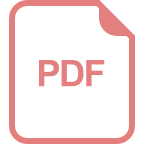
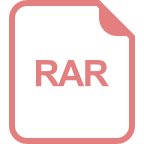
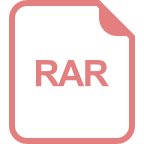













