score_dict = {}while True: input_str = input().strip() if input_str == '#': break name, score = input_str.split() score = int(score) if name in score_dict: score_dict[name].append(score) else: score_dict[name] = [score]print('姓 名 平均成绩')for name, scores in score_dict: avg_score = sum(scores) / len(scores) print('{:<8} {:.2f}'.format(name, avg_score))
时间: 2023-07-15 19:10:22 浏览: 54
这段代码存在一些问题,应该修改为:
```python
score_dict = {}
while True:
input_str = input().strip()
if input_str == '#':
break
name, score = input_str.split()
score = int(score)
if name in score_dict:
score_dict[name].append(score)
else:
score_dict[name] = [score]
print('姓 名 平均成绩')
for name, scores in score_dict.items():
avg_score = sum(scores) / len(scores)
print('{:<8} {:.2f}'.format(name, avg_score))
```
主要修改包括:
- 对于 `for name, scores in score_dict`,应该改为 `for name, scores in score_dict.items()`,因为字典类型需要使用 `items()` 方法来遍历。
- 对于计算平均成绩,应该在遍历时进行,而不是在输入时计算。
相关问题
def get_best_action_list(score_dict): best_action_list = [] max_score = MIN for key in score_dict: if max_score < score_dict[key]: best_action_list = [key] max_score = score_dict[key] elif max_score == score_dict[key]: best_action_list.append(key) return best_action_list
这是一个 Python 函数,接受一个字典参数 score_dict,返回一个列表,包含字典中值最大的键,如果有多个值相等的键,则都加入列表中。
其中 MIN 是一个常量,表示一个极小的数值,通常用于初始化 max_score 变量。函数的执行过程如下:
- 初始化 best_action_list 为空列表。
- 初始化 max_score 为 MIN。
- 对于字典中的每个键 key,如果该键对应的值 score_dict[key] 大于 max_score,则将 best_action_list 重置为 [key],并将 max_score 更新为 score_dict[key]。
- 如果该键对应的值 score_dict[key] 等于 max_score,则将该键加入 best_action_list 列表中。
- 返回 best_action_list 列表。
这个函数通常用于在多个可选项中选择最佳的选项,例如在游戏中选择最优策略,或者在推荐系统中选择最佳的推荐项。
while True: word_dict = {} fun_num = input('请输入功能编号:')
这是 Python 中的一段代码,它使用了一个 while 循环,表示程序会一直运行,直到用户选择退出。代码中的变量 word_dict 代表一个空的字典,用来存储用户添加的单词和其对应的释义。变量 fun_num 代表用户输入的功能编号,用来控制程序的不同功能。具体来说,程序会执行以下步骤:
1. 进入 while 循环,等待用户输入功能编号。
2. 用户输入功能编号后,程序将其存储在变量 fun_num 中,并进入 if 判断语句。
3. 根据用户输入的功能编号,程序执行相应的功能。例如,如果用户输入 1,程序会输出生词本中的所有单词及其释义;如果用户输入 2,程序会随机选择一个单词,并提示用户进行背诵。
4. 如果用户输入的是其他数字或非数字字符,程序会提示用户输入正确的功能编号,并继续等待用户输入。
5. 如果用户输入的是 0,表示用户想要退出程序,程序会跳出 while 循环,执行后续的代码,比如保存生词本到文件等。
总之,这段代码可以作为一个生词本程序的框架,实现基本的添加、查看、背诵和保存等功能。但具体的实现需要根据实际需求进行修改和完善。
相关推荐
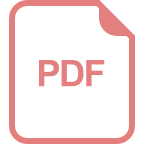
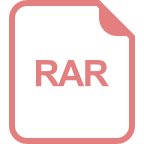













